用for语句编程实现:求n!,n的值从键盘输入
时间: 2023-05-20 13:07:04 浏览: 111
非常好的问题!以下是用for语句编程实现求n!的代码:
```
#include <stdio.h>
int main() {
int n, i;
long long factorial = 1;
printf("请输入一个正整数: ");
scanf("%d", &n);
// 如果输入的是负数,程序会提示错误
if (n < 0)
printf("错误!负数没有阶乘。");
else {
for (i = 1; i <= n; ++i) {
factorial *= i; // 阶乘计算
}
printf("%d 的阶乘 = %lld", n, factorial);
}
return 0;
}
```
希望这个代码能够帮到你!
相关问题
Python用for语句编程实现:求n!,n的值从键盘输入
可以使用以下代码实现:
n = int(input("请输入一个整数n:"))
result = 1
for i in range(1, n+1):
result *= i
print("%d的阶乘为:%d" % (n, result))
用c语言编程求n!,要求n从键盘输入。
好的,这个问题是关于编程的,我可以为您提供解答。下面是一个用C语言编写的求n!的代码:
```c
#include <stdio.h>
int main() {
int n, factorial = 1, i;
printf("请输入一个正整数n:");
scanf("%d", &n);
if (n < 0) {
printf("抱歉,您输入的不是正整数。\n");
return -1;
}
for (i = 1; i <= n; i++) {
factorial *= i;
}
printf("%d的阶乘是:%d\n", n, factorial);
return 0;
}
```
以上代码使用了for循环和条件判断语句,可以通过键盘输入一个正整数n,然后求n的阶乘并输出到屏幕上。如果输入的不是正整数,则会提示输入错误。希望能对您有所帮助!
相关推荐
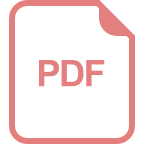
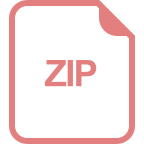












