c语言 实现睡觉的理发师问题(同步互斥方式采用信号量或mutex方式均可) 理发师问题的描述:一个理发店接待室有n张椅子,工作室有1张椅子;没有顾客时,理发师睡觉;第一个顾客来到时,必须将理发师唤醒;顾客来时如果还有空座的话,他就坐在一个座位上等待;如果顾客来时没有空座位了,他就离开,不理发了;当理发师处理完所有顾客,而又没有新顾客来时,他又开始睡觉。
时间: 2024-04-05 15:34:39 浏览: 114
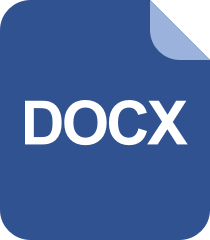
理发师多线程问题
下面是一个使用mutex方式实现的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define N 5 // 等候室中椅子的数量
int n = N; // 剩余椅子数量
int barber = 0; // 理发师是否在工作室中
int customers = 0; // 正在等待理发的顾客数量
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER; // 互斥锁
pthread_cond_t cond_customers = PTHREAD_COND_INITIALIZER; // 等待顾客的条件变量
pthread_cond_t cond_barber = PTHREAD_COND_INITIALIZER; // 等待理发师的条件变量
void* customer(void* arg) {
int id = *(int*)arg;
printf("Customer %d comes to the barber shop.\n", id);
pthread_mutex_lock(&mutex); // 获取互斥锁
if (n > 0) { // 等候室中有空椅子
n--;
printf("Customer %d sits down and waits.\n", id);
customers++;
pthread_cond_signal(&cond_customers); // 唤醒等待顾客的条件变量
pthread_mutex_unlock(&mutex); // 释放互斥锁
} else { // 等候室中没有空椅子
printf("No chairs available. Customer %d leaves.\n", id);
pthread_mutex_unlock(&mutex); // 释放互斥锁
return NULL;
}
pthread_mutex_lock(&mutex); // 获取互斥锁
while (!barber) { // 如果理发师在睡觉,等待理发师的条件变量
pthread_cond_wait(&cond_barber, &mutex);
}
printf("Customer %d enters the barber shop.\n", id);
customers--;
pthread_mutex_unlock(&mutex); // 释放互斥锁
// 理发师剪发
printf("Customer %d gets a haircut.\n", id);
return NULL;
}
void* barber(void* arg) {
while (1) { // 循环执行
pthread_mutex_lock(&mutex); // 获取互斥锁
if (customers == 0) { // 如果没有顾客,理发师睡觉
barber = 0;
printf("Barber goes to sleep.\n");
pthread_mutex_unlock(&mutex); // 释放互斥锁
} else { // 如果有顾客,理发师剪发
barber = 1;
printf("Barber wakes up and calls in the next customer.\n");
pthread_cond_signal(&cond_barber); // 唤醒等待理发师的条件变量
pthread_mutex_unlock(&mutex); // 释放互斥锁
// 理发师剪发
printf("Barber cuts hair.\n");
}
}
}
int main() {
pthread_t barber_thread;
pthread_t customer_threads[N];
int customer_ids[N];
// 创建理发师线程
pthread_create(&barber_thread, NULL, barber, NULL);
// 创建顾客线程
for (int i = 0; i < N; i++) {
customer_ids[i] = i + 1;
pthread_create(&customer_threads[i], NULL, customer, &customer_ids[i]);
sleep(1); // 等待1秒钟,模拟顾客到来的间隔时间
}
// 等待顾客线程结束
for (int i = 0; i < N; i++) {
pthread_join(customer_threads[i], NULL);
}
// 结束理发师线程
pthread_cancel(barber_thread);
pthread_join(barber_thread, NULL);
return 0;
}
```
这个代码示例中,我们使用了互斥锁和条件变量来实现同步。顾客线程尝试获取互斥锁,如果等候室中有空椅子,就占用一个椅子,并唤醒等待顾客的条件变量;否则,就离开理发店。当有顾客进入工作室时,理发师会剪发,直到所有顾客都被处理完毕。理发师线程循环执行,如果没有顾客,就睡觉;如果有顾客,就剪发。
阅读全文
相关推荐
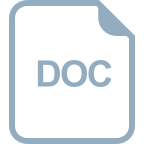
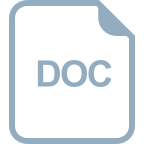





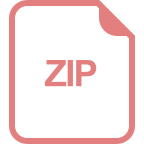
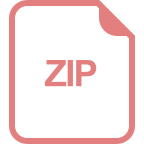
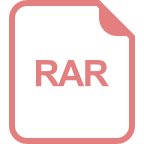
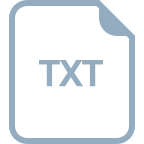
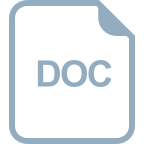
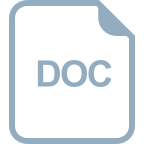
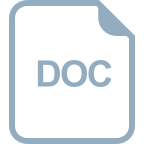
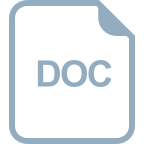


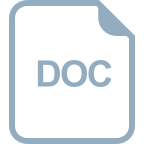