C语言用线程实现睡觉的理发师问题,(同步互斥方式采用信号量或mutex方式均可) 理发师问题的描述:一个理发店接待室有n张椅子,工作室有1张椅子;没有顾客时,理发师睡觉;第一个顾客来到时,必须将理发师唤醒;顾客来时如果还有空座的话,他就坐在一个座位上等待;如果顾客来时没有空座位了,他就离开,不理发了;当理发师处理完所有顾客,而又没有新顾客来时,他又开始睡觉。
时间: 2023-11-13 16:02:54 浏览: 60
好的,这是一个经典的线程同步问题。以下是C语言的代码实现,采用信号量方式进行同步互斥:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#include <unistd.h>
#define N 5 // 等候室座位数
#define M 1 // 工作室椅子数
sem_t customers; // 等候室中的顾客数目
sem_t barbers; // 工作室中的理发师数目
sem_t mutex; // 互斥锁,确保对座位的访问是互斥的
int waiting = 0; // 等候室中的等待人数
void *barber(void *arg)
{
while (1)
{
sem_wait(&customers); // 如果等候室中没有顾客,理发师要等待
sem_wait(&mutex); // 进入临界区
waiting--; // 有顾客进入了工作室,等待座位数减一
sem_post(&barbers); // 有一个理发师处于工作状态
sem_post(&mutex); // 退出临界区
// 理发师在工作
printf("Barber is working...\n");
sleep(3);
sem_wait(&mutex); // 进入临界区
sem_post(&customers); // 理发师完成工作,等待室中可以容纳一个顾客
sem_post(&mutex); // 退出临界区
}
}
void *customer(void *arg)
{
while (1)
{
sem_wait(&mutex); // 进入临界区
if (waiting < N) // 等候室中有空余座位
{
waiting++; // 有顾客来到等候室,等待人数加一
sem_post(&customers); // 顾客进入等候室
sem_post(&mutex); // 退出临界区
sem_wait(&barbers); // 等待理发师
printf("Customer is getting a haircut...\n");
sleep(2);
}
else // 等候室没有空余座位
{
sem_post(&mutex); // 退出临界区
printf("Customer is leaving...\n");
sleep(1);
}
}
}
int main()
{
pthread_t barber_thread, customer_thread;
sem_init(&customers, 0, 0);
sem_init(&barbers, 0, 0);
sem_init(&mutex, 0, 1);
// 创建理发师线程
if (pthread_create(&barber_thread, NULL, barber, NULL) != 0)
{
printf("Failed to create barber thread.\n");
exit(1);
}
// 创建顾客线程
if (pthread_create(&customer_thread, NULL, customer, NULL) != 0)
{
printf("Failed to create customer thread.\n");
exit(1);
}
// 等待线程结束
pthread_join(barber_thread, NULL);
pthread_join(customer_thread, NULL);
sem_destroy(&customers);
sem_destroy(&barbers);
sem_destroy(&mutex);
return 0;
}
```
相关推荐
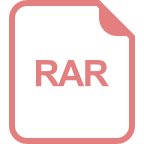
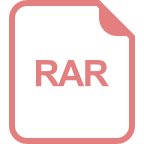
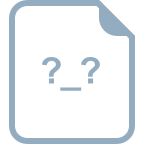














