用栈实现回文
时间: 2023-11-06 09:14:23 浏览: 50
好的,下面是一个用栈实现回文的示例代码,其中用到了栈类模板:
```c++
#include <iostream>
#include <stack>
#include <cstring>
using namespace std;
// 定义一个栈类模板
template<class type>
class Stack {
private:
type stack_1[1000];
int top;
public:
Stack() {
top = 0;
}
void push(type ob) {
stack_1[top++] = ob;
}
type pop() {
return stack_1[--top];
}
int empty() {
return top == 0;
}
};
// 判断一个字符串是否为回文字符串
bool isPalindrome(char* str) {
int n = strlen(str);
Stack<char> s;
for (int i = 0; i < n; i++) {
s.push(str[i]);
}
for (int i = 0; i < n; i++) {
if (s.pop() != str[i]) {
return false;
}
}
return true;
}
int main() {
char str[1000];
cout << "请输入一个字符串:" << endl;
cin >> str;
if (isPalindrome(str)) {
cout << str << " 是回文字符串。" << endl;
}
else {
cout << str << " 不是回文字符串。" << endl;
}
return 0;
}
```
在这个示例代码中,我们定义了一个栈类模板Stack,通过模板实例化出一个字符类型的栈s。在isPalindrome函数中,我们首先将字符串中的每一个字符入栈,然后再将栈中的每一个字符出栈,与原字符串中的字符比较,如果有不相等的字符,则不是回文字符串,返回false;否则是回文字符串,返回true。
希望这个示例代码对您有所帮助!
相关推荐
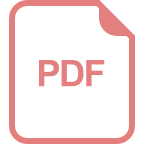
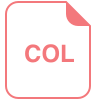
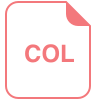












