#include<iostream> #include<cstdlib> #include <stack> #define max 1000 using namespace std; int main() { // read the datas int T, n; int W[max]; bool found = false; cout << "请输入背包大小: "; cin >> T; cout <<endl << "输入物品数量 : "; cin >> n; cout <<endl << "输入物品体积 : " <<endl; for (int j = 0; j < n; j++) cin >> W[j]; cout << endl << "解有 : " << endl; // process int left = T; stack<int> s; int i = 0; while (1) { if (left == W[i] || (left > W[i] && i < n)) { // 可以放进背包的情况 s.push(i); left -= W[i]; } if (left == 0) { // 刚好装满 // 标记 found = true; // 输出结果 stack<int> t; while (!s.empty()) { t.push( s.top()); s.pop(); } cout << "( "; while (!t.empty()) { cout << W[t.top()] << " "; s.push(t.top()); t.pop(); } cout << ")" <<endl; // 处理下一情况 if (s.size() == 1) { left += W[s.top()]; s.pop(); i++; if (i == n) break; } else if (i == n-1) { left += W[s.top()]; s.pop(); i = s.top() + 1; left += W[s.top()]; s.pop(); } else { i = s.top() + 1; left += W[s.top()]; s.pop(); } } else { // 不能装满 if (i == n-1) { // i遍历到最后一个物品 if (s.size() == 1 && s.top() == n - 1) { break; } else { if (i == s.top()) { left += W[s.top()]; s.pop(); i = s.top() + 1; left += W[s.top()];解释一下
时间: 2023-12-03 09:03:46 浏览: 21
这段代码是一个背包问题的求解程序,使用了回溯算法。程序首先读入背包的大小和物品数量,然后读入每个物品的体积。接着程序通过一个while循环,不断地进行回溯算法的搜索过程,找到所有能够放进背包的物品组合,并输出结果。
具体来说,程序使用了一个栈来保存当前已经放入背包中的物品编号,left变量表示当前背包剩余的容量。当可以放入一个物品时,将其编号压入栈中,并将left减去对应的体积。当背包被装满时,输出结果,并处理下一个情况。如果栈中只剩下一个物品,则说明已经遍历完了所有的情况,程序结束。否则,弹出栈中的两个物品,将左边的物品编号加1并更新left,进行下一轮搜索。
需要注意的是,这段代码没有对物品的价值进行考虑,仅仅是对物品体积的限制进行了处理。
相关问题
#include<cstdio> #include<cstdlib> #include<cstring> #include<cmath> #include<ctime> #include<iostream> #include<algorithm> #include<map> #include<stack> #include<queue> #include<vector> #include<set> #include<string> #define dd double #define ll long long dd PI = acos(-1); using namespace std; const ll MAXN = 1e5 + 5; const ll INF = 1e9 + 5; ll n; struct node { ll x, y; }s[5000]; ll dp[MAXN] = { 0 }; int main() { //ios::sync_with_stdio(false); ll n, m; cin >> n >> m; for (ll i = 1; i <= n; i++) { cin >> s[i].x >> s[i].y; } for (ll i = 1; i <= n; i++) { for (ll j = m; j >= s[i].x; j--) { dp[j] = max(dp[j], dp[j - s[i].x] + s[i].y); } } cout << dp[m] << endl; }
这是一段动态规划求解背包问题的代码,用来求解一个容量为m的背包能够装下的最大价值。具体实现过程为:
1. 定义一个结构体node,用来存储物品的重量和价值。
2. 读入物品数量n和背包容量m,然后读入每个物品的重量和价值。
3. 使用二重循环,遍历每一个物品,然后从大到小枚举背包容量j,更新dp[j]的值,表示容量为j的背包能够装下的最大价值。
4. 最后输出dp[m]的值,即容量为m的背包能够装下的最大价值。
值得注意的是,这段代码中还定义了一些常量和宏定义,如MAXN、INF等,以及一些头文件和命名空间的使用。
#include<iostream> #include<cstdlib> #include<cstdio> #include<cstring> #include<stack> using namespace std; #define MAXSIZE 301 int prior(char ch, bool flag) { if (ch == '+' || ch == '-') return 1; if (ch == '*' || ch == '/') return 2; if (ch == '(' && flag)return 0; if (ch == '(' && !flag)return 3; } int main() { string str; cin >> str; int i; char ch; stack<char>charact; for (i = 0; str[i] != '\0'; i++) { if ((str[i] <= 'z' && str[i] >= 'a') ||( str[i] <= 'Z' && str[i] >= 'A')) cout << str[i]; else if ( charact.empty()) { charact.push(str[i]); } else if (str[i] == '(')charact.push(str[i]); else if (str[i] == ')') { while (charact.top() != '('&&!charact.empty()) { ch = charact.top(); charact.pop(); cout << ch; }charact.pop(); } else if (str[i] == '+' || str[i] == '-' || str[i] == '*' || str[i] == '/') { while (prior(charact.top(), true) >= prior(str[i], false) && !charact.empty()) { ch = charact.top(); cout << ch; charact.pop(); }charact.push(str[i]); } }while (!charact.empty()) { ch = charact.top(); cout << ch; charact.pop(); } return 0; }
这是一个将中缀表达式转换为后缀表达式的代码,使用了栈的数据结构。具体思路是,从左到右扫描中缀表达式的每个元素,如果是操作数则直接输出,如果是运算符,则将其与栈顶元素比较优先级,如果优先级较低则将栈顶元素弹出并输出,直到栈顶元素优先级不低于当前运算符或者栈为空,然后将当前运算符入栈。如果是左括号则直接入栈,如果是右括号则将栈顶元素弹出并输出直到遇到左括号为止。
以下是代码解析和注释:
```c++
#include<iostream>
#include<cstdlib>
#include<cstdio>
#include<cstring>
#include<stack>
using namespace std;
#define MAXSIZE 301
// 判断运算符优先级的函数
int prior(char ch, bool flag) {
if (ch == '+' || ch == '-')
return 1;
if (ch == '*' || ch == '/')
return 2;
if (ch == '(' && flag) // 左括号优先级为0,用flag参数表示是否是当前运算符
return 0;
if (ch == '(' && !flag) // 如果是栈顶元素则优先级为3,因为栈顶元素优先级最低
return 3;
}
int main() {
string str;
cin >> str;
int i;
char ch;
stack<char> charact; // 存放运算符的栈
for (i = 0; str[i] != '\0'; i++) {
if ((str[i] <= 'z' && str[i] >= 'a') || (str[i] <= 'Z' && str[i] >= 'A')) // 如果是操作数则直接输出
cout << str[i];
else if (charact.empty()) // 如果栈为空则直接入栈
charact.push(str[i]);
else if (str[i] == '(') // 如果是左括号则直接入栈
charact.push(str[i]);
else if (str[i] == ')') { // 如果是右括号则弹出栈顶元素并输出直到遇到左括号为止
while (charact.top() != '(' && !charact.empty()) {
ch = charact.top();
charact.pop();
cout << ch;
}
charact.pop(); // 弹出左括号
} else if (str[i] == '+' || str[i] == '-' || str[i] == '*' || str[i] == '/') {
// 如果是运算符则比较优先级
while (prior(charact.top(), true) >= prior(str[i], false) && !charact.empty()) {
ch = charact.top();
cout << ch;
charact.pop();
}
charact.push(str[i]); // 将当前运算符入栈
}
}
// 扫描完后,将栈中剩余的运算符弹出并输出
while (!charact.empty()) {
ch = charact.top();
cout << ch;
charact.pop();
}
return 0;
}
```
相关推荐
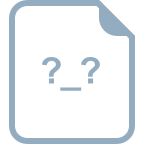







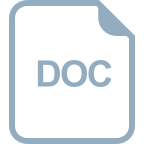
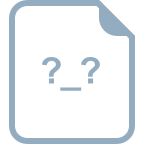
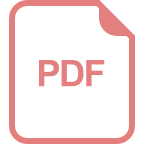
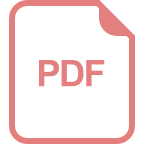