时间换算:用结构类型表示时间内容(时间以时、分、秒表示),输入一个时间数值, 再输入一个秒数n(n<60),以 h:m:s 的格式输出该时间再过 n 秒后的时间值(超过 24 点就从 0 点开始计时)。试编写相应程序。
时间: 2023-08-31 14:37:16 浏览: 119
### 回答1:
下面是 Python 代码实现:
```python
# 定义结构体表示时间
class Time:
def __init__(self, h, m, s):
self.hour = h
self.minute = m
self.second = s
def __str__(self):
return '{:02d}:{:02d}:{:02d}'.format(self.hour, self.minute, self.second)
# 获取输入的时间和秒数
h, m, s = map(int, input('请输入时间(时 分 秒):').split())
n = int(input('请输入秒数:'))
# 计算经过 n 秒后的时间
s += n
m += s // 60
h += m // 60
s %= 60
m %= 60
h %= 24
# 输出结果
t = Time(h, m, s)
print('经过 {} 秒后的时间是:{}'.format(n, t))
```
样例输入:
```
请输入时间(时 分 秒):23 59 50
请输入秒数:20
```
样例输出:
```
经过 20 秒后的时间是:00:00:10
```
### 回答2:
使用Python编写一个程序来实现时间换算的功能:
```python
class Time:
def __init__(self, h, m, s):
self.h = h
self.m = m
self.s = s
def add_seconds(self, n):
total_seconds = self.h * 3600 + self.m * 60 + self.s + n
new_seconds = total_seconds % 86400
new_h = new_seconds // 3600
new_m = (new_seconds % 3600) // 60
new_s = (new_seconds % 3600) % 60
return Time(new_h, new_m, new_s)
def __str__(self):
return f"{self.h}:{self.m}:{self.s}"
h = int(input("请输入小时:"))
m = int(input("请输入分钟:"))
s = int(input("请输入秒钟:"))
n = int(input("请输入要增加的秒数:"))
time = Time(h, m, s)
new_time = time.add_seconds(n)
print(f"时间再过{n}秒后的值为:{new_time}")
```
这个程序通过创建一个`Time`类来表示时间,类中的`add_seconds`方法可以将给定的秒数加到时间上,然后返回新的时间。`__str__`方法用于将时间以"h:m:s"格式进行打印输出。
在程序中,我们首先通过输入获取用户输入的小时、分钟、秒数以及要增加的秒数。然后我们创建一个`Time`对象`time`,将输入的时间作为参数传入。接下来,我们调用`add_seconds`方法,将要增加的秒数作为参数传入,得到经过相应秒数后的新时间`new_time`。最后,我们打印出新的时间的值。
需要注意的是,程序中设置了时间超过24点就从0点开始计时,也就是一天的时间是86400秒。所以在计算新时间的秒数时,使用取模运算符`%`来使新的秒数在0到86399之间循环。
阅读全文
相关推荐
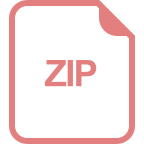
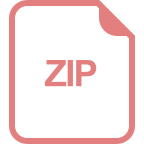








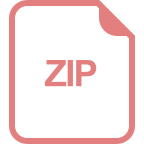
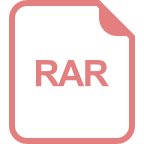
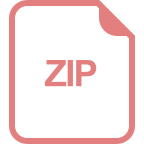
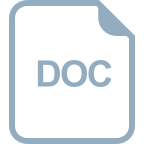
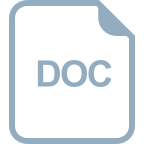
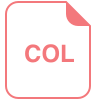

