IComparable 如果用C++怎么实现
时间: 2024-02-24 07:54:36 浏览: 108
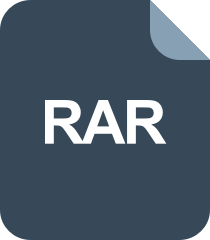
用c++语言编写的比较两个数的程序
在 C++ 中,可以通过定义一个抽象基类来实现 IComparable 接口的功能。具体实现方法如下:
1. 定义一个 IComparable 接口的抽象基类,并在其中声明纯虚的 CompareTo() 方法,用于比较对象。
```c++
class IComparable {
public:
virtual int CompareTo(const IComparable& other) const = 0;
};
```
2. 在需要进行排序的类中继承 IComparable 接口,并实现 CompareTo() 方法,用于比较对象。比较方法的实现可以根据实际需要进行修改。
```c++
class MyClass : public IComparable {
public:
int CompareTo(const IComparable& other) const override {
const MyClass& otherObj = dynamic_cast<const MyClass&>(other);
// 按照某种规则比较对象
if (this->value > otherObj.value) {
return 1;
} else if (this->value < otherObj.value) {
return -1;
} else {
return 0;
}
}
private:
int value;
};
```
3. 调用标准库中的 std::sort() 等排序算法进行排序操作,传入比较方法的实现。
```c++
std::vector<MyClass> vec;
// 添加 MyClass 对象
// ...
std::sort(vec.begin(), vec.end(), [](const MyClass& obj1, const MyClass& obj2) {
return obj1.CompareTo(obj2) < 0;
});
```
通过定义抽象基类和继承实现 IComparable 接口的方式,可以在 C++ 中实现类似于 C# 的 IComparable 接口的功能。
阅读全文
相关推荐
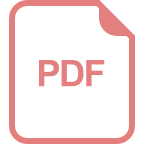
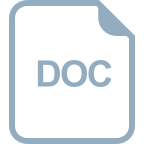

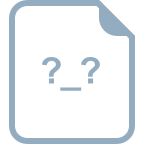
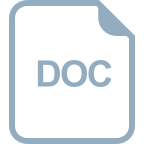
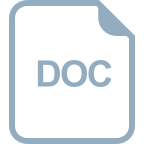
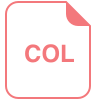
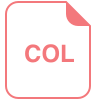

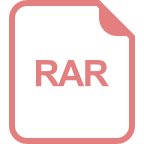
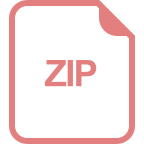
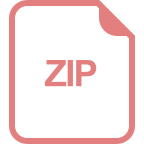
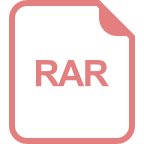
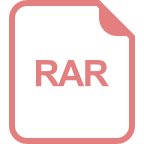
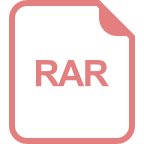
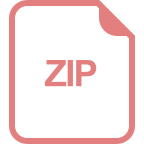
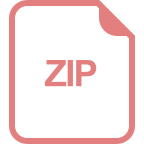
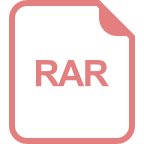