// 导入 countryNo string[] buf = File.ReadAllLines(textBox_CountryNo.Text); string[] buf2 = File.ReadAllLines(textBox_CityNo.Text); foreach (string line in buf) { string[] ss = line.Split('\t'); if (ss.Length < 2) continue; try { byte index = byte.Parse(ss[0], System.Globalization.NumberStyles.AllowHexSpecifier); if (!myCountryNoDict.ContainsKey(index)) { myCountryNoDict.Add(index, ss[1]); } } catch (Exception ee) { } } for (int ii = 0; ii < buf2.Length; ++ii) { myCityNoDict.Add(ii, buf2[ii]); } // 导入 countryValue int valueFileLen = (int)new FileInfo(textBox_CountryValue.Text).Length; if (valueFileLen != ipCCount) { Console.WriteLine(" Must Choose legal countryValue file !!!!"); return; } int valueFile2Len = (int)new FileInfo(textBox_CityValue.Text).Length; if (valueFileLen != ipCCount) { Console.WriteLine(" Must Choose legal cityValue file !!!!"); return; } using (FileStream fs = File.OpenRead(textBox_CountryValue.Text)) { fs.Read(countryValueBuf, 0, ipCCount); } // 验证countryValue与countryNo 文件是否冲突 foreach (byte vv in countryValueBuf) { if (!myCountryNoDict.ContainsKey(vv)) { Console.WriteLine(" CountryValue conflict CountryNo!!!"); ViewClear(); return; } } 转java
时间: 2024-02-10 10:26:03 浏览: 27
以下是将给定的代码片段转换为Java的等效代码:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.HashMap;
public class CountryCityImport {
public static void main(String[] args) {
String[] buf;
String[] buf2;
HashMap<Byte, String> myCountryNoDict = new HashMap<>();
HashMap<Integer, String> myCityNoDict = new HashMap<>();
int ipCCount = 0;
byte[] countryValueBuf;
try {
buf = Files.readAllLines(Paths.get(textBox_CountryNo.getText())).toArray(new String[0]);
buf2 = Files.readAllLines(Paths.get(textBox_CityNo.getText())).toArray(new String[0]);
for (String line : buf) {
String[] ss = line.split("\t");
if (ss.length < 2)
continue;
try {
byte index = Byte.parseByte(ss[0], 16);
if (!myCountryNoDict.containsKey(index)) {
myCountryNoDict.put(index, ss[1]);
}
} catch (NumberFormatException e) {
// Handle exception
}
}
for (int ii = 0; ii < buf2.length; ++ii) {
myCityNoDict.put(ii, buf2[ii]);
}
Path countryValuePath = Paths.get(textBox_CountryValue.getText());
Path cityValuePath = Paths.get(textBox_CityValue.getText());
int valueFileLen = (int) Files.size(countryValuePath);
int valueFile2Len = (int) Files.size(cityValuePath);
if (valueFileLen != ipCCount) {
System.out.println(" Must Choose legal countryValue file !!!!");
return;
}
if (valueFile2Len != ipCCount) {
System.out.println(" Must Choose legal cityValue file !!!!");
return;
}
countryValueBuf = Files.readAllBytes(countryValuePath);
// 验证countryValue与countryNo文件是否冲突
for (byte vv : countryValueBuf) {
if (!myCountryNoDict.containsKey(vv)) {
System.out.println(" CountryValue conflict CountryNo!!!");
ViewClear();
return;
}
}
} catch (IOException e) {
// Handle exception
}
}
private static void ViewClear() {
// Implement the logic to clear the view
}
}
```
请注意,上述代码中的`textBox_CountryNo.getText()`,`textBox_CityNo.getText()`,`textBox_CountryValue.getText()`和`textBox_CityValue.getText()`需要替换为实际的文本框或输入字段的逻辑。另外,`ViewClear()`方法需要根据实际需求进行实现。
相关推荐
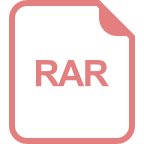
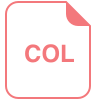















