vue从本地存储中获取登录用户的信息,具体代码
时间: 2024-02-20 11:59:09 浏览: 26
可以使用`localStorage`来存储用户信息,然后在Vue中获取并使用。下面是一个示例代码:
```javascript
// 存储用户信息
localStorage.setItem('user', JSON.stringify({
name: 'Alice',
age: 20
}))
// 获取用户信息
const user = JSON.parse(localStorage.getItem('user'))
// 在Vue组件中使用用户信息
export default {
data() {
return {
user: {}
}
},
mounted() {
this.user = JSON.parse(localStorage.getItem('user'))
}
}
```
在上面的代码中,我们先使用`localStorage`存储了一个名为`user`的用户信息对象,然后在Vue组件中获取并使用该对象。在组件的`mounted()`生命周期函数中,我们从`localStorage`中获取`user`对象,并将其赋值给组件的`data`属性中的`user`变量,这样我们就可以在组件中使用该变量了。当然,如果需要修改用户信息,也可以将修改后的对象重新存储到`localStorage`中。
相关问题
vue获取登录页面用户信息
以下是获取登录用户信息的Vue代码示例:
```javascript
// 从localStorage中获取用户信息
let name = localStorage.getItem("name");
console.log(name);
// 从cookie中获取用户信息
import { getCookie } from 'src/js/cookieUtil'
let userInfo = getCookie('userInfo');
console.log(userInfo);
```
在上述代码中,我们首先使用`localStorage.getItem()`方法从本地存储中获取用户信息,然后使用`console.log()`方法将其打印出来。接下来,我们使用`import`语句导入了一个名为`cookieUtil`的模块,并使用其中的`getCookie()`方法从cookie中获取用户信息。最后,我们再次使用`console.log()`方法将其打印出来。
vue获取用户信息
获取用户信息的方式可以有很多种,具体取决于你的系统架构和需求。以下是一些常见的方法:
1. 在用户登录时,将用户信息存储在本地存储或会话存储中。当需要获取用户信息时,直接从本地存储或会话存储中读取。
2. 在前端使用JWT(JSON Web Token)来验证用户身份,并附带一些用户信息。当需要获取用户信息时,解码JWT即可。
3. 在前端发送请求时,将用户token作为请求头附带,后端在接收到请求后解析token,获取用户信息并返回。
下面是一个示例代码,使用第二种方式获取用户信息:
```javascript
import jwtDecode from 'jwt-decode';
// 假设用户token已经存储在localStorage中
const token = localStorage.getItem('token');
// 解码token,获取用户信息
const userInfo = jwtDecode(token);
console.log(userInfo);
```
需要注意的是,由于JWT是基于对称加密的,因此不要在JWT中存储敏感信息,例如密码等。
相关推荐
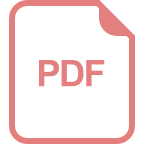
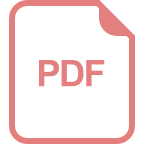
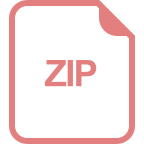
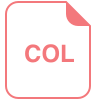
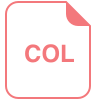
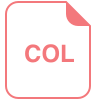
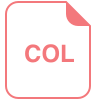
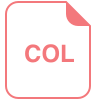







