使用selenium爬取网页表格
时间: 2023-03-19 15:22:53 浏览: 141
使用 Selenium 可以模拟浏览器操作,包括打开网页、点击按钮、输入文本、获取网页源代码等。因此,使用 Selenium 可以方便地爬取网页中的表格数据。
以下是使用 Selenium 爬取网页表格的一般步骤:
1. 导入必要的库
```python
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
```
2. 创建浏览器对象
```python
driver = webdriver.Chrome()
```
这里选择使用 Chrome 浏览器,需要先下载 ChromeDriver。
3. 打开网页
```python
url = "http://www.example.com"
driver.get(url)
```
4. 找到表格元素
可以使用 Selenium 提供的 find_element_by_* 方法找到表格元素,例如:
```python
table = driver.find_element_by_id("table_id")
```
5. 获取表格数据
获取表格数据有多种方式,以下是其中两种:
(1)遍历表格行和列,逐一获取单元格数据:
```python
rows = table.find_elements_by_tag_name("tr")
for row in rows:
cols = row.find_elements_by_tag_name("td")
for col in cols:
print(col.text)
```
(2)将表格数据读取到 Pandas DataFrame 中:
```python
import pandas as pd
html_table = driver.find_element_by_xpath("//table")
df_list = pd.read_html(html_table.get_attribute('outerHTML'))
df = df_list[0]
```
6. 关闭浏览器
```python
driver.quit()
```
完整的代码示例:
```python
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
import pandas as pd
# 创建浏览器对象
driver = webdriver.Chrome()
# 打开网页
url = "http://www.example.com"
driver.get(url)
# 找到表格元素
table = driver.find_element_by_id("table_id")
# 将表格数据读取到 Pandas DataFrame 中
html_table = driver.find_element_by_xpath("//table")
df_list = pd.read_html(html_table.get_attribute('outerHTML'))
df = df_list[0]
# 关闭浏览器
driver.quit()
```
相关推荐
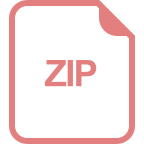
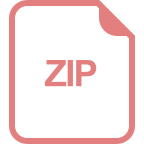














