爬取亚马逊对应前十产品图片以及相应的ASIN、评分、价格、小类目、排名、链接,并保存到excel表格中
时间: 2023-08-31 22:58:03 浏览: 267
要实现这个功能,你可以使用Selenium和BeautifulSoup库来爬取亚马逊的产品信息,并使用Pandas库将数据保存到Excel表格中。下面是一个示例代码:
```python
from selenium import webdriver
from selenium.webdriver.common.by import By
from bs4 import BeautifulSoup
import pandas as pd
# 设置Chrome浏览器的路径
chrome_path = "path_to_chrome_driver"
# 创建一个WebDriver实例
driver = webdriver.Chrome(chrome_path)
# 打开亚马逊网站
driver.get("https://www.amazon.com")
# 定位搜索框并输入搜索关键词
search_box = driver.find_element(By.ID, "twotabsearchtextbox")
search_box.send_keys("your search keyword")
search_box.submit()
# 等待页面加载完成
driver.implicitly_wait(10)
# 获取前十个产品的信息
product_list = driver.find_elements(By.CSS_SELECTOR, ".s-result-item")
data = []
for product in product_list[:10]:
soup = BeautifulSoup(product.get_attribute("innerHTML"), "html.parser")
# 解析产品信息
asin = soup.find("div", {"data-asin": True})["data-asin"]
image = soup.find("img")["src"]
title = soup.find("span", class_="a-text-normal").get_text(strip=True)
rating = soup.find("span", class_="a-icon-alt").get_text(strip=True)
price = soup.find("span", class_="a-offscreen").get_text(strip=True)
category = soup.find("span", class_="a-size-base a-color-secondary").get_text(strip=True)
rank = soup.find("span", class_="s-compact-format").get_text(strip=True)
link = "https://www.amazon.com" + soup.find("a", class_="a-link-normal")["href"]
# 将产品信息添加到列表中
data.append({
"ASIN": asin,
"Image": image,
"Title": title,
"Rating": rating,
"Price": price,
"Category": category,
"Rank": rank,
"Link": link
})
# 关闭浏览器
driver.quit()
# 将数据保存到Excel表格中
df = pd.DataFrame(data)
df.to_excel("amazon_products.xlsx", index=False)
```
请将`path_to_chrome_driver`替换为你的Chrome驱动程序的路径。此代码将搜索结果的前十个产品的ASIN、图片链接、标题、评分、价格、小类目、排名和链接保存到名为`amazon_products.xlsx`的Excel表格中。你可以根据需求调整代码以获取更多的产品信息或修改保存的文件名。
阅读全文
相关推荐
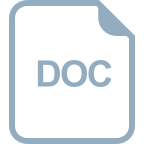
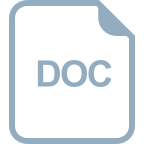
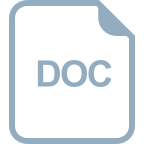

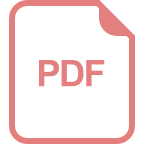

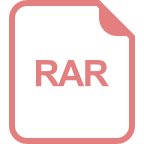
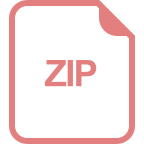
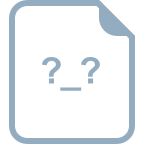
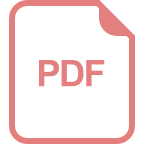
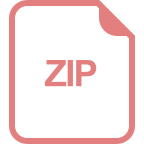
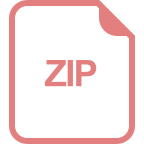
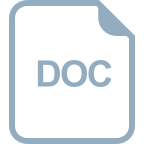
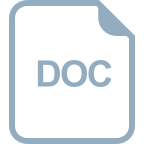
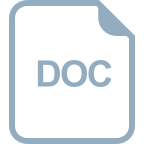
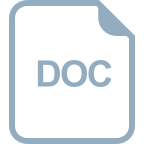

