用python写贪吃蛇代码
时间: 2023-09-26 12:07:53 浏览: 52
好的,我可以回答这个问题。以下是 Python 语言的贪吃蛇游戏代码示例:
``` python
import pygame
from pygame.locals import *
import random
pygame.init()
# 设置游戏窗口尺寸
window_width = 480
window_height = 480
window = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption('贪吃蛇')
# 定义颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
blue = (0, 0, 255)
green = (0, 255, 0)
# 设置字体
font_style = pygame.font.SysFont(None, 30)
# 定义蛇身和食物的大小
block_size = 10
# 记录分数
def score(score):
value = font_style.render("Score: " + str(score), True, white)
window.blit(value, [0, 0])
# 画出蛇的长度
def snake(block_size, snakeList):
for x in snakeList:
pygame.draw.rect(window, green, [x[0], x[1], block_size, block_size])
# 提示文字
def message(msg, color):
mesg = font_style.render(msg, True, color)
window.blit(mesg, [window_width / 6, window_height / 3])
# 开始游戏
def gameLoop():
game_over = False
game_close = False
# 蛇的初始位置
x1 = window_width / 2
y1 = window_height / 2
# 蛇每次移动的位置
x1_change = 0
y1_change = 0
# 蛇身列表
snakeList = []
Length_of_snake = 1
# 食物位置
foodx = round(random.randrange(0, window_width - block_size) / 10.0) * 10.0
foody = round(random.randrange(0, window_height - block_size) / 10.0) * 10.0
while not game_over:
while game_close == True:
window.fill(black)
message("You Lost! Q-Quit or C-Play Again", red)
score(Length_of_snake - 1)
pygame.display.update()
for event in pygame.event.get():
if event.type == KEYDOWN:
if event.key == K_q:
game_over = True
game_close = False
if event.key == K_c:
gameLoop()
for event in pygame.event.get():
if event.type == QUIT:
game_over = True
if event.type == KEYDOWN:
if event.key == K_LEFT:
x1_change = -block_size
y1_change = 0
elif event.key == K_RIGHT:
x1_change = block_size
y1_change = 0
elif event.key == K_UP:
y1_change = -block_size
x1_change = 0
elif event.key == K_DOWN:
y1_change = block_size
x1_change = 0
if x1 >= window_width or x1 < 0 or y1 >= window_height or y1 < 0:
game_close = True
x1 += x1_change
y1 += y1_change
window.fill(black)
# 画出食物
pygame.draw.rect(window, blue, [foodx, foody, block_size, block_size])
# 生成蛇身
snake_Head = []
snake_Head.append(x1)
snake_Head.append(y1)
snakeList.append(snake_Head)
if len(snakeList) > Length_of_snake:
del snakeList[0]
# 判断是否吃到了食物
for eachSegment in snakeList[:-1]:
if eachSegment == snake_Head:
game_close = True
# 绘制蛇身
snake(block_size, snakeList)
score(Length_of_snake - 1)
pygame.display.update()
if x1 == foodx and y1 == foody:
foodx = round(random.randrange(0, window_width - block_size) / 10.0) * 10.0
foody = round(random.randrange(0, window_height - block_size) / 10.0) * 10.0
Length_of_snake += 1
pygame.display.update()
pygame.quit()
quit()
gameLoop()
```
希望这可以帮到你!
相关推荐
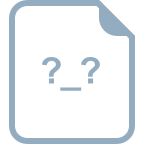
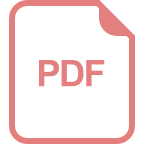
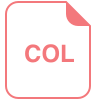
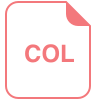
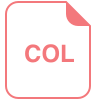
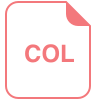
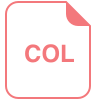









