用Python 写贪吃蛇
时间: 2023-11-19 21:21:09 浏览: 30
好的,以下是一个简单的 Python 贪吃蛇游戏的示例代码:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 设置游戏窗口的大小
window_width = 500
window_height = 500
game_window = pygame.display.set_mode((window_width, window_height))
# 设置游戏标题
pygame.display.set_caption('贪吃蛇')
# 定义颜色
black = (0, 0, 0)
white = (255, 255, 255)
red = (255, 0, 0)
# 定义蛇的初始位置和大小
snake_block_size = 10
snake_list = []
snake_length = 1
snake_x = window_width / 2
snake_y = window_height / 2
# 定义食物的初始位置和大小
food_block_size = 10
food_x = round(random.randrange(0, window_width - food_block_size) / 10.0) * 10.0
food_y = round(random.randrange(0, window_height - food_block_size) / 10.0) * 10.0
# 定义蛇的移动方向和速度
snake_direction = 'RIGHT'
snake_speed = 10
# 定义字体
font_style = pygame.font.SysFont(None, 30)
# 定义显示文字的函数
def message(msg, color):
text = font_style.render(msg, True, color)
game_window.blit(text, [window_width / 6, window_height / 3])
# 游戏循环
game_over = False
while not game_over:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
snake_direction = 'LEFT'
elif event.key == pygame.K_RIGHT:
snake_direction = 'RIGHT'
elif event.key == pygame.K_UP:
snake_direction = 'UP'
elif event.key == pygame.K_DOWN:
snake_direction = 'DOWN'
# 移动蛇
if snake_direction == 'RIGHT':
snake_x += snake_speed
elif snake_direction == 'LEFT':
snake_x -= snake_speed
elif snake_direction == 'UP':
snake_y -= snake_speed
elif snake_direction == 'DOWN':
snake_y += snake_speed
# 判断是否吃到食物
if snake_x == food_x and snake_y == food_y:
food_x = round(random.randrange(0, window_width - food_block_size) / 10.0) * 10.0
food_y = round(random.randrange(0, window_height - food_block_size) / 10.0) * 10.0
snake_length += 1
# 绘制蛇和食物
game_window.fill(black)
pygame.draw.rect(game_window, red, [food_x, food_y, food_block_size, food_block_size])
snake_head = []
snake_head.append(snake_x)
snake_head.append(snake_y)
snake_list.append(snake_head)
if len(snake_list) > snake_length:
del snake_list[0]
for x in snake_list[:-1]:
if x == snake_head:
game_over = True
for block in snake_list:
pygame.draw.rect(game_window, white, [block[0], block[1], snake_block_size, snake_block_size])
pygame.display.update()
# 判断是否撞墙
if snake_x >= window_width or snake_x < 0 or snake_y >= window_height or snake_y < 0:
game_over = True
# 显示分数
score = snake_length - 1
score_text = font_style.render("Score: " + str(score), True, white)
game_window.blit(score_text, [0, 0])
# 更新屏幕
pygame.display.update()
# 退出 Pygame
pygame.quit()
```
运行代码后,你将会看到一个简单的贪吃蛇游戏窗口。通过键盘上的方向键来控制蛇的移动方向,尽可能地吃到食物并躲避撞墙和撞到自己的情况。
相关推荐
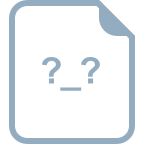
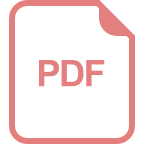
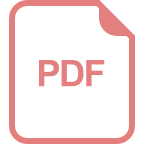
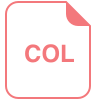
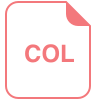
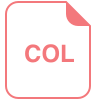
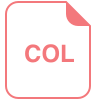
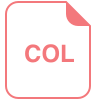







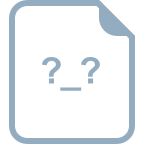