帮我设计一个程序,从键盘上输入15个单词,将其以降序方式显示出来(单词不得雷同)
时间: 2024-03-26 12:41:05 浏览: 47
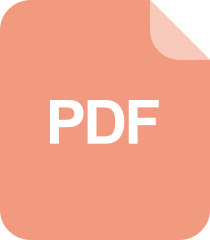
基于Blended Learning混合式教学在程序设计课程上的开发.pdf
以下是一个简单的 C 语言程序,可以实现你的要求:
```c
#include <stdio.h>
#include <string.h>
#define MAX_WORDS 15
#define MAX_LENGTH 20
void sort_words(char words[][MAX_LENGTH], int n);
void print_words(char words[][MAX_LENGTH], int n);
int main() {
char words[MAX_WORDS][MAX_LENGTH];
int count = 0;
// 从键盘上输入单词,如果输入的单词已经存在,则忽略
while (count < MAX_WORDS) {
char new_word[MAX_LENGTH];
printf("Enter word #%d: ", count + 1);
scanf("%s", new_word);
int i;
for (i = 0; i < count; i++) {
if (strcmp(words[i], new_word) == 0) {
printf("Word already exists, please enter a new word.\n");
break;
}
}
if (i >= count) {
strcpy(words[count], new_word);
count++;
}
}
// 对单词进行排序,并输出
sort_words(words, count);
print_words(words, count);
return 0;
}
/**
* 对单词进行排序,使用简单的选择排序算法
*/
void sort_words(char words[][MAX_LENGTH], int n) {
int i, j;
for (i = 0; i < n - 1; i++) {
int max_index = i;
for (j = i + 1; j < n; j++) {
if (strcmp(words[j], words[max_index]) > 0) {
max_index = j;
}
}
if (max_index != i) {
char temp[MAX_LENGTH];
strcpy(temp, words[i]);
strcpy(words[i], words[max_index]);
strcpy(words[max_index], temp);
}
}
}
/**
* 输出单词,每个单词之间用空格隔开
*/
void print_words(char words[][MAX_LENGTH], int n) {
printf("Sorted words (in descending order):\n");
int i;
for (i = 0; i < n; i++) {
printf("%s ", words[i]);
}
printf("\n");
}
```
这个程序使用了一个二维字符数组 `words` 来存储用户输入的单词,使用 `strcmp` 函数判断输入的单词是否重复,使用选择排序算法对单词进行降序排序。最后输出排序后的单词,每个单词之间用空格隔开。
阅读全文
相关推荐
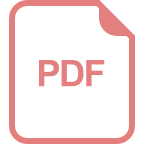
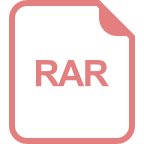















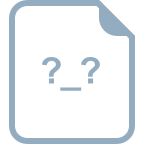