利用哈希表的构造方法建立一个小型的信息管理系统,实现插入,查找,删除,计数,输出等功能,并能在屏幕上输出相应的结果
时间: 2023-05-28 13:04:35 浏览: 141
以下是一个基于哈希表的小型信息管理系统的示例代码:
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
const int TABLE_SIZE = 1000;
class Person {
public:
string name;
int age;
string phone;
Person() {}
Person(const string& name, int age, const string& phone) : name(name), age(age), phone(phone) {}
};
class HashTable {
private:
vector<vector<Person>> table;
int hash(const string& key) {
int sum = 0;
for (char c : key) {
sum += static_cast<int>(c);
}
return sum % TABLE_SIZE;
}
public:
HashTable() {
table.resize(TABLE_SIZE);
}
void insert(const Person& p) {
int index = hash(p.name);
table[index].push_back(p);
}
bool search(const string& name, Person& p) {
int index = hash(name);
for (const auto& person : table[index]) {
if (person.name == name) {
p = person;
return true;
}
}
return false;
}
bool remove(const string& name) {
int index = hash(name);
auto& bucket = table[index];
auto it = find_if(bucket.begin(), bucket.end(), [&](const Person& p) { return p.name == name; });
if (it != bucket.end()) {
bucket.erase(it);
return true;
}
return false;
}
int count() {
int count = 0;
for (const auto& bucket : table) {
count += bucket.size();
}
return count;
}
void print() {
for (const auto& bucket : table) {
for (const auto& person : bucket) {
cout << "Name: " << person.name << ", Age: " << person.age << ", Phone: " << person.phone << endl;
}
}
}
};
int main() {
HashTable ht;
bool running = true;
while (running) {
cout << "1. Insert\n2. Search\n3. Remove\n4. Count\n5. Print\n6. Quit\nEnter your choice: ";
int choice;
cin >> choice;
switch (choice) {
case 1: {
string name, phone;
int age;
cout << "Enter name: ";
cin >> name;
cout << "Enter age: ";
cin >> age;
cout << "Enter phone: ";
cin >> phone;
ht.insert(Person(name, age, phone));
break;
}
case 2: {
string name;
cout << "Enter name: ";
cin >> name;
Person p;
if (ht.search(name, p)) {
cout << "Name: " << p.name << ", Age: " << p.age << ", Phone: " << p.phone << endl;
} else {
cout << "Person not found" << endl;
}
break;
}
case 3: {
string name;
cout << "Enter name: ";
cin >> name;
if (ht.remove(name)) {
cout << "Person removed" << endl;
} else {
cout << "Person not found" << endl;
}
break;
}
case 4: {
cout << "Number of people: " << ht.count() << endl;
break;
}
case 5: {
ht.print();
break;
}
case 6: {
running = false;
break;
}
default: {
cout << "Invalid choice" << endl;
break;
}
}
}
return 0;
}
在这个示例代码中,我们使用一个哈希表来存储人员信息,每个桶存储一个 vector,其中包含具有相同哈希值的人员信息。我们使用一个简单的哈希函数,将每个人的姓名转换为一个整数索引,然后将其插入到相应的桶中。
该程序提供了以下操作:
1. 插入:提示用户输入人员姓名、年龄和电话号码,然后将其插入哈希表中。
2. 查找:提示用户输入人员姓名,然后在哈希表中查找该人员并输出其详细信息。
3. 删除:提示用户输入人员姓名,然后从哈希表中删除该人员。
4. 计数:输出哈希表中包含的人员数量。
5. 输出:按顺序输出哈希表中所有人员的详细信息。
6. 退出:终止程序。
这个示例程序可以根据需要进行扩展,例如增加其他属性(如地址、电子邮件等)、实现更复杂的搜索操作(如按年龄、电话等搜索)、使用更复杂的哈希函数等。
阅读全文
相关推荐

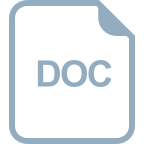
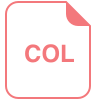
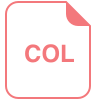
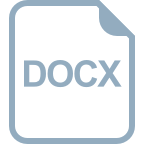
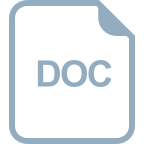
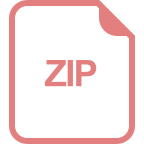
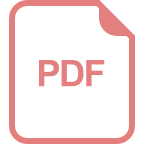
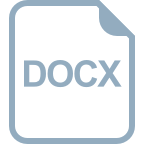
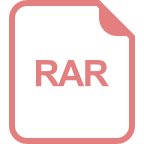
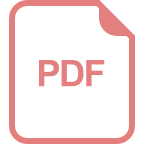