3、桌上有个能盛得下五个水果的空盘子。爸爸不停地向盘中放苹果或桔子,儿子 不停地从盘中取出桔子,女儿不停地从盘中取出苹果。规定三人不能同时从盘中取 放水果。试用信号量实现爸爸、儿子和女儿这三个进程之间的同步。(10分)请用c语言
时间: 2024-12-01 11:29:12 浏览: 54
在C语言中,我们可以使用互斥量(mutex)和信号量(semaphore)来模拟这种场景中的同步。这里,我们需要三个互斥量(mutex)分别代表苹果、桔子和盘子状态,以及两个信号量(semaphore)用于表示剩余的水果数量。
首先,定义两个全局信号量,`apple_semaphore` 和 `orange_semaphore` 分别初始化为5,表示初始有5个苹果和5个橘子。另一个全局互斥量 `plate_mutex` 保证只有一个进程可以操作盘子。
```c
#include <pthread.h>
#include <semaphore.h>
#define MAX_APPLES 5
#define MAX_ORANGES 5
sem_t apple_semaphore = SEM_VALUE_MAX;
sem_t orange_semaphore = SEM_VALUE_MAX;
pthread_mutex_t plate_mutex = PTHREAD_MUTEX_INITIALIZER;
void* dad_produce(void* arg);
void* son_consume_apples(void* arg);
void* daughter_consume_oranges(void* arg);
int main() {
// 创建生产者(爸爸)线程
pthread_t dad_thread, son_thread, daughter_thread;
pthread_create(&dad_thread, NULL, dad_produce, NULL);
pthread_create(&son_thread, NULL, son_consume_apples, NULL);
pthread_create(&daughter_thread, NULL, daughter_consume_oranges, NULL);
// 等待所有线程结束
pthread_join(dad_thread, NULL);
pthread_join(son_thread, NULL);
pthread_join(daughter_thread, NULL);
return 0;
}
// 生产者(爸爸)函数
void* dad_produce(void* arg) {
for (int i = 0; i < MAX_APPLES; i++) {
pthread_mutex_lock(&plate_mutex);
sem_wait(&apple_semaphore); // 当盘子里没苹果时等待
printf("爸爸往盘子里放了一个苹果\n");
sem_post(&apple_semaphore); // 水果增加,唤醒其他消费者
pthread_mutex_unlock(&plate_mutex);
}
return NULL;
}
// 消费者(儿子)函数
void* son_consume_apples(void* arg) {
while (true) {
pthread_mutex_lock(&plate_mutex);
if (sem_wait(&apple_semaphore) == -1 && errno == EDEADLK) break; // 盘子里没苹果就退出
printf("儿子拿走了一个苹果\n");
sem_wait(&orange_semaphore); // 取苹果前确认盘子里有橘子
sem_post(&apple_semaphore);
pthread_mutex_unlock(&plate_mutex);
}
return NULL;
}
// 消费者(女儿)函数
void* daughter_consume_oranges(void* arg) {
while (true) {
pthread_mutex_lock(&plate_mutex);
if (sem_wait(&orange_semaphore) == -1 && errno == EDEADLK) break; // 盘子里没橘子就退出
printf("女儿拿走了一个橘子\n");
sem_wait(&apple_semaphore); // 取橘子前确认盘子里有苹果
sem_post(&orange_semaphore);
pthread_mutex_unlock(&plate_mutex);
}
return NULL;
}
```
在这个例子中,每个消费者(儿子和女儿)在取水果之前都会检查信号量,如果当前无法获取所需的水果,它们会释放自己占用的资源并等待。当爸爸添加新的水果时,他会先获取互斥量,然后减小相应的信号量值,唤醒等待的消费者。
阅读全文
相关推荐


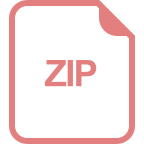













