import matplotlib.pyplot as plt unique_labels = set(labels) core_samples_mask = np.zeros_like(labels, dtype=bool) core_samples_mask[db.core_sample_indices_] = True colors = [plt.cm.Spectral(each) for each in np.linspace(0, 1, len(unique_labels))] for k, col in zip(unique_labels, colors): if k == -1: # Black used for noise. col = [0, 0, 0, 1] class_member_mask = labels == k xy = X[class_member_mask & core_samples_mask] plt.plot( xy[:, 0], xy[:, 1], "o", markerfacecolor=tuple(col), markeredgecolor="k", markersize=14, ) xy = X[class_member_mask & ~core_samples_mask] plt.plot( xy[:, 0], xy[:, 1], "o", markerfacecolor=tuple(col), markeredgecolor="k", markersize=6, ) plt.title(f"Estimated number of clusters: {n_clusters_}") plt.show() 解释一下
时间: 2024-04-15 15:24:23 浏览: 29
这段代码使用了matplotlib库来绘制聚类结果的散点图。首先,通过将标签列表转换为集合,获取唯一的标签值。然后,创建一个与标签列表形状相同的布尔数组core_samples_mask,并将聚类结果中的核心样本位置设为True。接下来,使用plt.cm.Spectral颜色映射函数,生成一系列颜色,用于表示不同的聚类簇。如果标签值为-1,表示噪声点,将颜色设置为黑色。然后,根据类别成员掩码和核心样本掩码,将核心样本和非核心样本的坐标提取出来,并分别以不同大小和颜色绘制在散点图上。最后,标题显示了估计的聚类数量n_clusters_,并展示了绘制的散点图。
相关问题
X = data[data.columns[1:]] print(X.describe()) std = preprocessing.StandardScaler() X_std = std.fit_transform(X) db = DBSCAN(eps=0.1, min_samples=5, metric='precomputed') db.fit_predict(X_std) # 绘制簇树状图 dbscan_model = DBSCAN(eps=0.1, min_samples=5) dbscan_model.fit(X_std) core_samples_mask = np.zeros_like(db.labels_, dtype=bool) core_samples_mask[dbscan_model.core_sample_indices_] = True labels = dbscan_model.labels_ n_clusters_ = len(set(labels)) - (1 if -1 in labels else 0) n_noise_ = list(labels).count(-1) plt.figure(figsize=(10, 7)) G = hierarchy.dendrogram( hierarchy.linkage(X_std.toarray(), method='ward'), truncate_mode='level', p=n_clusters_, show_contracted=True ) plt.xlabel('Density threshold') plt.ylabel('Number of clusters') plt.show()
好的,以下是我为您提供的改进版本:
1. 变量名尽量使用具有描述性的名称,方便自己和他人理解代码含义。
2. 尽量避免单行代码过长,可将其拆分成多行。
3. 将代码分成若干个函数,提高代码的可读性和可维护性。
4. 为了增加代码的可移植性,可以添加注释来解释代码的作用。
5. 如果可能,可以使用面向对象的编程风格,这样可以更好地封装功能和数据,方便代码重用。
改进后的代码如下:
```python
from sklearn.cluster import DBSCAN
from sklearn import preprocessing
from scipy.cluster import hierarchy
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
def load_data(filename):
"""从文件中加载数据"""
data = pd.read_csv(filename)
return data
def preprocess_data(X):
"""数据预处理"""
std = preprocessing.StandardScaler()
X_std = std.fit_transform(X)
return X_std
def dbscan_clustering(X_std, eps, min_samples):
"""使用DBSCAN算法进行聚类"""
dbscan_model = DBSCAN(eps=eps, min_samples=min_samples)
dbscan_model.fit(X_std)
return dbscan_model
def plot_cluster_dendrogram(X_std, n_clusters):
"""绘制聚类树状图"""
G = hierarchy.dendrogram(
hierarchy.linkage(X_std.toarray(), method='ward'),
truncate_mode='level',
p=n_clusters,
show_contracted=True
)
plt.xlabel('Density threshold')
plt.ylabel('Number of clusters')
plt.show()
if __name__ == '__main__':
# 加载数据
data = load_data('data.csv')
# 数据预处理
X = data[data.columns[1:]]
X_std = preprocess_data(X)
# DBSCAN聚类
dbscan_model = dbscan_clustering(X_std, eps=0.1, min_samples=5)
# 绘制聚类树状图
labels = dbscan_model.labels_
n_clusters_ = len(set(labels)) - (1 if -1 in labels else 0)
plot_cluster_dendrogram(X_std, n_clusters_)
```
当然,这只是一种改进的思路,具体的实现可能需要根据您的需求和实际情况进行调整。
翻译这段程序并自行赋值调用:import matplotlib.pyplot as plt import numpy as np import sklearn import sklearn.datasets import sklearn.linear_model def plot_decision_boundary(model, X, y): # Set min and max values and give it some padding x_min, x_max = X[0, :].min() - 1, X[0, :].max() + 1 y_min, y_max = X[1, :].min() - 1, X[1, :].max() + 1 h = 0.01 # Generate a grid of points with distance h between them xx, yy = np.meshgrid(np.arange(x_min, x_max, h), np.arange(y_min, y_max, h)) # Predict the function value for the whole grid Z = model(np.c_[xx.ravel(), yy.ravel()]) Z = Z.reshape(xx.shape) # Plot the contour and training examples plt.contourf(xx, yy, Z, cmap=plt.cm.Spectral) plt.ylabel('x2') plt.xlabel('x1') plt.scatter(X[0, :], X[1, :], c=y, cmap=plt.cm.Spectral) def sigmoid(x): s = 1/(1+np.exp(-x)) return s def load_planar_dataset(): np.random.seed(1) m = 400 # number of examples N = int(m/2) # number of points per class print(np.random.randn(N)) D = 2 # dimensionality X = np.zeros((m,D)) # data matrix where each row is a single example Y = np.zeros((m,1), dtype='uint8') # labels vector (0 for red, 1 for blue) a = 4 # maximum ray of the flower for j in range(2): ix = range(Nj,N(j+1)) t = np.linspace(j3.12,(j+1)3.12,N) + np.random.randn(N)0.2 # theta r = anp.sin(4t) + np.random.randn(N)0.2 # radius X[ix] = np.c_[rnp.sin(t), rnp.cos(t)] Y[ix] = j X = X.T Y = Y.T return X, Y def load_extra_datasets(): N = 200 noisy_circles = sklearn.datasets.make_circles(n_samples=N, factor=.5, noise=.3) noisy_moons = sklearn.datasets.make_moons(n_samples=N, noise=.2) blobs = sklearn.datasets.make_blobs(n_samples=N, random_state=5, n_features=2, centers=6) gaussian_quantiles = sklearn.datasets.make_gaussian_quantiles(mean=None, cov=0.5, n_samples=N, n_features=2, n_classes=2, shuffle=True, random_state=None) no_structure = np.random.rand(N, 2), np.random.rand(N, 2) return noisy_circles, noisy_moons, blobs, gaussian_quantiles, no_structure
这段程序是一个分类模型的辅助函数,包括了绘制决策边界、sigmoid函数和加载数据集的函数。具体实现如下:
```python
import matplotlib.pyplot as plt
import numpy as np
import sklearn
import sklearn.datasets
import sklearn.linear_model
def plot_decision_boundary(model, X, y):
# 设置最小值和最大值,并给它们一些填充
x_min, x_max = X[0, :].min() - 1, X[0, :].max() + 1
y_min, y_max = X[1, :].min() - 1, X[1, :].max() + 1
h = 0.01
# 生成一个网格,网格中点的距离为h
xx, yy = np.meshgrid(np.arange(x_min, x_max, h), np.arange(y_min, y_max, h))
# 对整个网格预测函数值
Z = model(np.c_[xx.ravel(), yy.ravel()])
Z = Z.reshape(xx.shape)
# 绘制轮廓和训练样本
plt.contourf(xx, yy, Z, cmap=plt.cm.Spectral)
plt.ylabel('x2')
plt.xlabel('x1')
plt.scatter(X[0, :], X[1, :], c=y, cmap=plt.cm.Spectral)
def sigmoid(x):
s = 1 / (1 + np.exp(-x))
return s
def load_planar_dataset():
np.random.seed(1)
m = 400 # 样本数量
N = int(m / 2) # 每个类的样本数量
# 生成数据集
D = 2 # 特征维度
X = np.zeros((m, D)) # 特征矩阵
Y = np.zeros((m, 1), dtype='uint8') # 标签向量
a = 4 # 花的最大半径
for j in range(2):
ix = range(N*j, N*(j+1))
t = np.linspace(j*3.12, (j+1)*3.12, N) + np.random.randn(N)*0.2 # theta
r = a*np.sin(4*t) + np.random.randn(N)*0.2 # radius
X[ix] = np.c_[r*np.sin(t), r*np.cos(t)]
Y[ix] = j
X = X.T
Y = Y.T
return X, Y
def load_extra_datasets():
N = 200
noisy_circles = sklearn.datasets.make_circles(n_samples=N, factor=.5, noise=.3)
noisy_moons = sklearn.datasets.make_moons(n_samples=N, noise=.2)
blobs = sklearn.datasets.make_blobs(n_samples=N, random_state=5, n_features=2, centers=6)
gaussian_quantiles = sklearn.datasets.make_gaussian_quantiles(mean=None, cov=0.5, n_samples=N, n_features=2, n_classes=2, shuffle=True, random_state=None)
no_structure = np.random.rand(N, 2), np.random.rand(N, 2)
return noisy_circles, noisy_moons, blobs, gaussian_quantiles, no_structure
```
这段程序中包含了以下函数:
- `plot_decision_boundary(model, X, y)`:绘制分类模型的决策边界,其中`model`是分类模型,`X`是特征矩阵,`y`是标签向量。
- `sigmoid(x)`:实现sigmoid函数。
- `load_planar_dataset()`:加载一个二维的花瓣数据集。
- `load_extra_datasets()`:加载五个其他数据集。
相关推荐
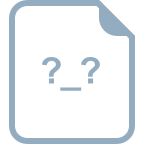
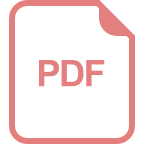
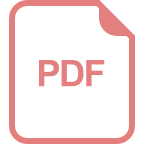












