java设置JFrame的事件,不穿透到JButton
时间: 2024-05-04 08:18:07 浏览: 10
您可以在JButton上添加一个鼠标监听器,以防止事件穿透到JFrame。以下是一个示例代码:
```
button.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
// 防止事件穿透
e.consume();
// 在这里添加您的按钮事件处理代码
}
});
```
在这个示例中,我们添加了一个鼠标单击事件监听器,如果单击了按钮,它将阻止事件穿透到JFrame,并执行您的按钮事件处理代码。
相关问题
java设置JFrame的移动事件,不穿透到JButton
要实现这个功能,你可以在JFrame中添加一个MouseListener来监听鼠标事件,并在mousePressed()方法中记录鼠标点击时的坐标。然后在mouseDragged()方法中计算出鼠标移动的距离,并将JFrame的位置相应地移动。
以下是一个示例代码:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class MyFrame extends JFrame {
private int mouseX, mouseY;
private JButton button;
public MyFrame() {
super("Move JFrame");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 400);
// 添加一个按钮来测试
button = new JButton("Click me!");
add(button, BorderLayout.CENTER);
// 添加MouseListener来监听鼠标事件
addMouseListener(new MouseAdapter() {
public void mousePressed(MouseEvent e) {
// 记录鼠标点击时的坐标
mouseX = e.getX();
mouseY = e.getY();
}
});
// 添加MouseMotionListener来监听鼠标移动事件
addMouseMotionListener(new MouseAdapter() {
public void mouseDragged(MouseEvent e) {
// 计算鼠标移动的距离,并将JFrame位置相应地移动
int deltaX = e.getX() - mouseX;
int deltaY = e.getY() - mouseY;
setLocation(getLocation().x + deltaX, getLocation().y + deltaY);
}
});
}
public static void main(String[] args) {
MyFrame frame = new MyFrame();
frame.setVisible(true);
}
}
```
在这个示例中,我们创建了一个MyFrame类来继承JFrame,并添加了一个按钮和两个MouseListener和MouseMotionListener来监听鼠标事件。在mousePressed()方法中,我们记录了鼠标点击时的坐标,而在mouseDragged()方法中,我们计算了鼠标移动的距离,并将JFrame的位置相应地移动。由于按钮没有添加MouseListener,所以鼠标事件不会穿透到按钮上。
java 设置JFrame大小
Java设置JFrame大小的方法有多种,常见的有:
1. 使用setSize()方法设置JFrame的宽和高,例如:frame.setSize(500, 400);
2. 使用setPreferredSize()方法设置JFrame的首选大小,例如:frame.setPreferredSize(new Dimension(500, 400));
3. 使用pack()方法自动调整JFrame的大小以适应其内容,例如:frame.pack();
4. 使用setResizable()方法设置JFrame是否可调整大小,例如:frame.setResizable(false)。
希望我的回答有帮助到您。
相关推荐
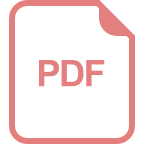












