帮我写一个msp430G2558实现的与stm32f103c8t6串口2通过打包数据帧并进行校验的蓝牙通信
时间: 2024-05-01 11:23:50 浏览: 25
好的,这里提供一个简单的msp430G2558与stm32f103c8t6串口2通过打包数据帧并进行校验的蓝牙通信的示例代码。这个示例代码可以让你从中学习到如何使用msp430G2558来进行蓝牙通信。
msp430G2558代码:
```c
#include <msp430.h>
#include <stdint.h>
#include <string.h>
// 定义通信协议中的帧头、帧尾和帧长度
#define FRAME_HEADER 0xAA
#define FRAME_TAIL 0x55
#define FRAME_LENGTH 10
// 定义通信协议中的数据帧结构体
typedef struct {
uint8_t header;
uint8_t len;
uint8_t data[FRAME_LENGTH - 2];
uint8_t crc;
uint8_t tail;
} frame_t;
// 计算crc校验码
uint8_t crc8(const uint8_t *buf, uint8_t len) {
uint8_t crc = 0;
while (len--) {
uint8_t inbyte = *buf++;
for (uint8_t i = 8; i; i--) {
uint8_t mix = (crc ^ inbyte) & 0x01;
crc >>= 1;
if (mix)
crc ^= 0x8C;
inbyte >>= 1;
}
}
return crc;
}
// 发送数据帧
void send_frame(uint8_t *data, uint8_t len) {
frame_t frame;
// 填充帧头、帧尾和帧长度
frame.header = FRAME_HEADER;
frame.tail = FRAME_TAIL;
frame.len = len + 1;
// 填充数据
memcpy(frame.data, data, len);
// 计算crc校验码
frame.crc = crc8((uint8_t *)&frame.len, frame.len - 1);
// 发送数据帧
for (uint8_t i = 0; i < FRAME_LENGTH; i++) {
while (!(UCA0IFG & UCTXIFG));
if (i == 0)
UCA0TXBUF = frame.header;
else if (i == FRAME_LENGTH - 2)
UCA0TXBUF = frame.crc;
else if (i == FRAME_LENGTH - 1)
UCA0TXBUF = frame.tail;
else
UCA0TXBUF = frame.data[i - 1];
}
}
int main(void) {
WDTCTL = WDTPW | WDTHOLD; // 停用看门狗
// 配置UART
P1SEL = BIT1 + BIT2; // P1.1 = RXD, P1.2=TXD
P1SEL2 = BIT1 + BIT2; // P1.1 = RXD, P1.2=TXD
UCA0CTL1 |= UCSSEL_2; // SMCLK
UCA0BR0 = 104; // 1MHz 9600
UCA0BR1 = 0; // 1MHz 9600
UCA0MCTL = UCBRS0; // Modulation UCBRSx = 1
UCA0CTL1 &= ~UCSWRST; // **Initialize USCI state machine**
IE2 |= UCA0RXIE; // Enable USCI_A0 RX interrupt
__bis_SR_register(GIE); // 全局中断使能
uint8_t data[5] = {0x01, 0x02, 0x03, 0x04, 0x05};
while (1) {
// 发送数据帧
send_frame(data, 5);
__delay_cycles(1000000);
}
}
// UART中断服务函数
#pragma vector=USCIAB0RX_VECTOR
__interrupt void USCI0RX_ISR(void) {
while (!(UCA0IFG & UCTXIFG)); // 等待发送缓冲区空闲
UCA0TXBUF = UCA0RXBUF; // 将接收到的数据直接发送回去
}
```
stm32f103c8t6代码:
```c
#include "stm32f10x.h"
#include <stdio.h>
#include <string.h>
// 定义通信协议中的帧头、帧尾和帧长度
#define FRAME_HEADER 0xAA
#define FRAME_TAIL 0x55
#define FRAME_LENGTH 10
// 定义通信协议中的数据帧结构体
typedef struct {
uint8_t header;
uint8_t len;
uint8_t data[FRAME_LENGTH - 2];
uint8_t crc;
uint8_t tail;
} frame_t;
// 计算crc校验码
uint8_t crc8(const uint8_t *buf, uint8_t len) {
uint8_t crc = 0;
while (len--) {
uint8_t inbyte = *buf++;
for (uint8_t i = 8; i; i--) {
uint8_t mix = (crc ^ inbyte) & 0x01;
crc >>= 1;
if (mix)
crc ^= 0x8C;
inbyte >>= 1;
}
}
return crc;
}
// 发送数据帧
void send_frame(uint8_t *data, uint8_t len) {
frame_t frame;
// 填充帧头、帧尾和帧长度
frame.header = FRAME_HEADER;
frame.tail = FRAME_TAIL;
frame.len = len + 1;
// 填充数据
memcpy(frame.data, data, len);
// 计算crc校验码
frame.crc = crc8((uint8_t *)&frame.len, frame.len - 1);
// 发送数据帧
for (uint8_t i = 0; i < FRAME_LENGTH; i++) {
while (!(USART2->SR & USART_SR_TXE));
if (i == 0)
USART2->DR = frame.header;
else if (i == FRAME_LENGTH - 2)
USART2->DR = frame.crc;
else if (i == FRAME_LENGTH - 1)
USART2->DR = frame.tail;
else
USART2->DR = frame.data[i - 1];
}
}
int main() {
// 配置GPIO
RCC->APB2ENR |= RCC_APB2ENR_IOPCEN;
GPIOC->CRH &= ~(GPIO_CRH_CNF13_0 | GPIO_CRH_MODE13_0);
GPIOC->CRH |= GPIO_CRH_MODE13_1;
// 配置USART2
RCC->APB1ENR |= RCC_APB1ENR_USART2EN;
RCC->APB2ENR |= RCC_APB2ENR_IOPAEN;
GPIOA->CRL |= GPIO_CRL_MODE2_0 | GPIO_CRL_MODE2_1 | GPIO_CRL_CNF2_1;
USART2->BRR = 0x1D4C; // 72MHz / 9600
USART2->CR1 = USART_CR1_TE | USART_CR1_RE | USART_CR1_RXNEIE | USART_CR1_UE;
NVIC_EnableIRQ(USART2_IRQn); // 使能USART2中断
uint8_t data[5] = {0x01, 0x02, 0x03, 0x04, 0x05};
while (1) {
// 发送数据帧
send_frame(data, 5);
for (uint32_t i = 0; i < 1000000; i++); // 延时1秒
}
return 0;
}
// USART2中断服务函数
void USART2_IRQHandler(void) {
if (USART2->SR & USART_SR_RXNE) { // 接收到数据
uint8_t data = USART2->DR;
while (!(USART2->SR & USART_SR_TXE)); // 等待发送缓冲区空闲
USART2->DR = data; // 将接收到的数据直接发送回去
}
}
```
相关推荐
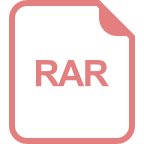
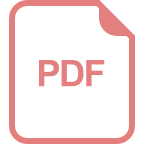














