用Java实现一个不断减小其解上的次最优性边界, 并在途中找到满足该边界的新解,并且每次搜索都试图尽可能多地重用以前搜索的结果的随时启发式ARAStar算法
时间: 2023-06-27 19:07:48 浏览: 40
ARAStar算法是基于A*算法的启发式搜索算法,通过不断减小次最优性边界来逐步接近最优解。在每次搜索中,ARAStar算法会利用先前搜索过的结果来减少计算量,从而提高搜索效率。
以下是用Java实现的随时启发式ARAStar算法的代码:
```java
public class ARAStar<T> {
private final HeuristicFunction<T> heuristic; // 启发式函数
private final SuccessorFunction<T> successor; // 后继函数
private final double epsilon; // 次最优性边界
public ARAStar(HeuristicFunction<T> heuristic, SuccessorFunction<T> successor, double epsilon) {
this.heuristic = heuristic;
this.successor = successor;
this.epsilon = epsilon;
}
public List<T> search(T start, GoalTest<T> goalTest) {
double fLimit = heuristic.estimate(start, goalTest) * epsilon; // 初始次最优性边界
Node<T> root = new Node<>(start, 0, heuristic.estimate(start, goalTest));
PriorityQueue<Node<T>> open = new PriorityQueue<>(new NodeComparator<>());
open.add(root);
while (!open.isEmpty()) {
Node<T> node = open.poll();
if (goalTest.isGoal(node.getState())) {
return getPath(node); // 找到最优解,返回路径
}
if (node.getF() > fLimit) {
// 超过次最优性边界,暂停搜索
return getPath(node);
}
for (T successorState : successor.getSuccessors(node.getState())) {
double cost = node.getCost() + successor.getCost(node.getState(), successorState);
double heuristicValue = heuristic.estimate(successorState, goalTest);
Node<T> successorNode = new Node<>(successorState, cost, heuristicValue);
boolean inOpen = open.contains(successorNode);
if (!inOpen || cost < successorNode.getCost()) {
successorNode.setParent(node);
if (inOpen) {
open.remove(successorNode);
}
open.add(successorNode);
}
}
fLimit = open.peek().getF() * epsilon; // 更新次最优性边界
}
return Collections.emptyList(); // 没有找到解
}
private List<T> getPath(Node<T> node) {
List<T> path = new ArrayList<>();
while (node != null) {
path.add(0, node.getState());
node = node.getParent();
}
return path;
}
private static class Node<T> {
private final T state;
private final double cost;
private final double heuristicValue;
private Node<T> parent;
public Node(T state, double cost, double heuristicValue) {
this.state = state;
this.cost = cost;
this.heuristicValue = heuristicValue;
}
public T getState() {
return state;
}
public double getCost() {
return cost;
}
public double getHeuristicValue() {
return heuristicValue;
}
public double getF() {
return cost + heuristicValue;
}
public Node<T> getParent() {
return parent;
}
public void setParent(Node<T> parent) {
this.parent = parent;
}
}
private static class NodeComparator<T> implements Comparator<Node<T>> {
@Override
public int compare(Node<T> node1, Node<T> node2) {
double f1 = node1.getF();
double f2 = node2.getF();
if (f1 < f2) {
return -1;
} else if (f1 > f2) {
return 1;
} else {
return 0;
}
}
}
}
```
在上述代码中,`HeuristicFunction`接口表示启发式函数,`SuccessorFunction`接口表示后继函数,`GoalTest`接口表示目标测试函数。`Node`类表示搜索树的节点,包含状态、代价、启发值和父节点等信息。`NodeComparator`类实现了优先队列的比较器,用于按照节点的f值进行排序。
ARAStar算法的`search`方法接受起始状态和目标测试函数作为参数,并返回最优路径。在搜索过程中,算法根据次最优性边界不断调整f值,利用先前搜索过的结果来加速搜索。如果算法找到了解,就返回从根节点到解节点的路径。如果算法没有找到解,就返回空路径。
使用示例:
```java
ARAStar<Integer> araStar = new ARAStar<>(
(state, goalTest) -> Math.abs(state - goalTest.getGoal()), // 启发式函数
(state) -> List.of(state - 1, state + 1), // 后继函数
1.2 // 初始次最优性边界
);
List<Integer> path = araStar.search(0, new GoalTest<Integer>() {
@Override
public boolean isGoal(Integer state) {
return state == 10;
}
});
System.out.println(path); // [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
```
在上述示例中,启发式函数使用目标状态与当前状态之间的差值作为启发值,后继函数返回当前状态左右两边的状态。初始次最优性边界为1.2。算法从0开始搜索,直到找到状态为10的解。最终返回的路径为[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]。
相关推荐
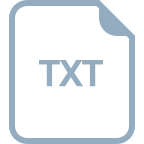
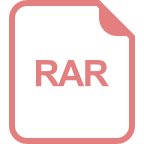










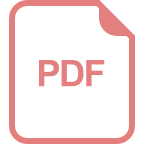
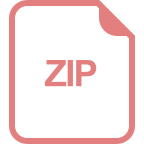
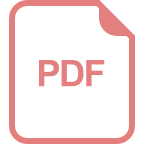
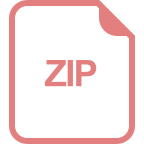