设置LinkList的头指针怎么设置
时间: 2024-09-28 10:03:09 浏览: 22
在C++中,如果你正在创建一个简单的单向链表(如`LinkedList`),并想要设置它的头指针(通常称为`head`),你需要完成以下步骤:
1. **首先**,定义一个链表节点的数据结构(Node)。每个节点包含一个数据成员(`data`)和一个指向下一个节点的指针(`next`):
```cpp
struct Node {
int data;
Node* next; // 指向下一个节点的指针
};
```
2. **其次**,如果链表为空(即没有节点),你可以直接将`head`设置为`nullptr`表示空链表:
```cpp
Node* head = nullptr;
```
3. **如果**你想添加第一个节点到链表,那么需要创建一个新的节点并将其`next`字段设为`nullptr`,然后设置`head`指向新节点:
```cpp
Node* newNode = new Node();
newNode->data = /* 要存储的数据 */;
newNode->next = nullptr;
head = newNode;
```
4. **如果链表已有节点**,当你添加新的节点作为头部时,也只需修改`head`的指向:
```cpp
// 假设你已经有了一个现有节点p
p->next = newNode;
head = newNode;
```
这表明`newNode`现在成为了链表的新开始(头部)。
注意,记得在不再需要节点时,手动释放内存以防止内存泄漏:
```cpp
delete newNode;
```
相关问题
linklist双指针列表反转java
链表双指针法是一种常用的链表操作技巧,常用于反转链表。在Java中,你可以通过两个指向链表节点的指针,一个指向当前节点(通常称为`prev`),另一个指向下一个节点(`curr`),然后逐步交换它们的指向来进行反转。以下是简单的步骤:
1. 初始化两个指针,`prev`设为`null`,`curr`设为链表头节点。
2. 当`curr`不为空时,执行以下操作:
- 将`curr`的下一个节点(`next`)保存到临时变量中。
- 更新`curr`的下一个节点为`prev`。
- 移动`prev`和`curr`分别向前一位,即`prev = curr`, `curr = next`。
3. 当`curr`变为`null`时,说明已经到达了原链表的尾部,此时`prev`就是新的头节点。
这是Java代码的一个简单实现:
```java
public ListNode reverseList(ListNode head) {
ListNode prev = null;
ListNode curr = head;
while (curr != null) {
ListNode nextTemp = curr.next; // 保存当前节点的下一个节点
curr.next = prev; // 将当前节点的next指向前一个节点
prev = curr; // 指针前移
curr = nextTemp; // 指针再次前移
}
return prev; // 返回新链表的头节点
}
```
LinkList locate_LinkList(LinkList H, char key[]); //H为单链表的头指针,key为要查询的姓名
你好!谢谢你的问题。根据你给出的函数原型,这个函数是用来查询某个姓名在单链表中的位置的。具体实现方法可能类似于遍历链表,逐一比对姓名,直到找到目标姓名为止。如果找到了,则返回该节点位置的指针;如果没有找到,则返回一个空指针。
请注意,我的回答只是基于你的问题中提供的信息给出的一个简要概述。如果你需要更多的细节或者有其他问题,请继续提问,我会尽力为你解答。同时,我也会遵守你的要求,不会透露你提出的任何问题或要求。
阅读全文
相关推荐
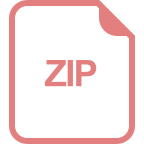
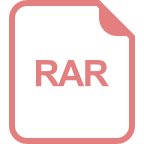
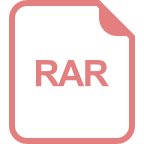
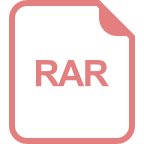













