(1)编写html页面,并使用ajax请求显示html页面内容。 获取数据 (2)请完成下面的任务:使用javascirpt编写程序,请求JSON网页。
时间: 2023-06-20 22:08:58 浏览: 102
(1)以下是一个使用ajax请求并显示HTML页面内容的示例:
```html
<!DOCTYPE html>
<html>
<head>
<title>Ajax请求HTML页面示例</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id="btn">加载HTML页面</button>
<div id="content"></div>
<script>
$(document).ready(function(){
$("#btn").click(function(){
$.ajax({
url: "example.html",
success: function(data){
$("#content").html(data); // 将获取到的HTML代码插入到页面中
}
});
});
});
</script>
</body>
</html>
```
这个示例中,我们使用了jQuery库来简化ajax请求操作。当用户点击“加载HTML页面”按钮时,我们使用ajax请求获取example.html页面的内容,并将其插入到id为“content”的div中。
(2)以下是一个使用javascript请求JSON网页的示例:
```html
<!DOCTYPE html>
<html>
<head>
<title>Ajax请求JSON网页示例</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id="btn">请求JSON数据</button>
<div id="content"></div>
<script>
$(document).ready(function(){
$("#btn").click(function(){
$.getJSON("example.json", function(data){
$("#content").html(JSON.stringify(data)); // 将获取到的JSON数据转换成字符串并插入到页面中
});
});
});
</script>
</body>
</html>
```
这个示例中,当用户点击“请求JSON数据”按钮时,我们使用getJSON方法请求example.json文件的内容,并将其转换成字符串后插入到id为“content”的div中。注意,$.getJSON方法已经将响应的数据自动解析成了一个JavaScript对象,因此我们可以直接使用data变量来访问其属性。
阅读全文
相关推荐
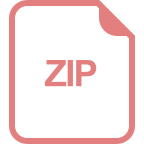
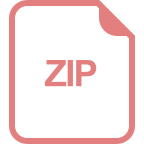
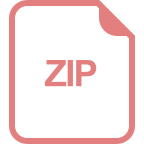















