用python写一个帧差法目标跟踪算法
时间: 2023-08-22 19:10:09 浏览: 82
好的,以下是使用Python实现帧差法目标跟踪算法的基本步骤:
1. 导入必要的库和模块:
```python
import cv2
import numpy as np
```
2. 初始化摄像头并读取第一帧:
```python
cap = cv2.VideoCapture(0)
ret, frame = cap.read()
```
3. 将第一帧转换为灰度图像:
```python
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
```
4. 循环处理每一帧图像:
```python
while True:
# 读取当前帧
ret, frame = cap.read()
# 转换为灰度图像
gray_frame = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 计算前一帧和当前帧的差异
diff = cv2.absdiff(gray, gray_frame)
# 对差异图像进行二值化处理
threshold = cv2.threshold(diff, 25, 255, cv2.THRESH_BINARY)[1]
# 对二值化图像进行膨胀操作
kernel = np.ones((5, 5), np.uint8)
dilation = cv2.dilate(threshold, kernel, iterations=2)
# 查找轮廓并绘制矩形框
contours, hierarchy = cv2.findContours(dilation, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
if cv2.contourArea(contour) < 2000:
continue
(x, y, w, h) = cv2.boundingRect(contour)
cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2)
# 显示结果
cv2.imshow("Frame", frame)
cv2.imshow("Threshold", threshold)
# 更新前一帧图像
gray = gray_frame
# 按下 q 键退出循环
if cv2.waitKey(1) & 0xFF == ord('q'):
break
```
在这个代码中,我们使用 `cv2.absdiff` 计算前一帧和当前帧的差异,然后使用 `cv2.threshold` 对差异图像进行二值化处理,再使用 `cv2.dilate` 对二值化图像进行膨胀操作,最后使用 `cv2.findContours` 查找轮廓并绘制矩形框来跟踪目标。
注意:该算法只能跟踪静止的目标,不能跟踪移动的目标。如果需要跟踪移动的目标,可以使用更高级的算法,比如卡尔曼滤波。
阅读全文
相关推荐
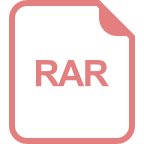
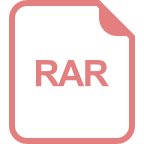







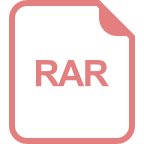
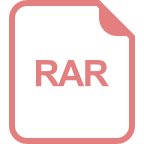
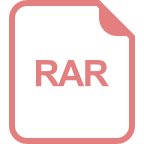
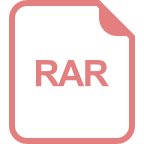
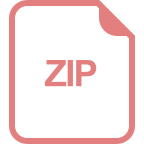
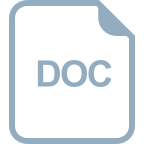


