用html和javascripe写一个贪吃蛇小游戏
时间: 2023-05-17 12:05:49 浏览: 174
好的,以下是一个简单的贪吃蛇小游戏的 HTML 和 JavaScript 代码:
HTML 代码:
```
<!DOCTYPE html>
<html>
<head>
<title>贪吃蛇小游戏</title>
<style>
canvas {
border: 1px solid black;
}
</style>
</head>
<body>
<canvas id="canvas" width="400" height="400"></canvas>
<script src="snake.js"></script>
</body>
</html>
```
JavaScript 代码(保存为 snake.js 文件):
```
var canvas = document.getElementById("canvas");
var ctx = canvas.getContext("2d");
var snake = [{x: 10, y: 10}];
var food = {x: 0, y: 0};
var direction = "right";
var score = 0;
function drawSnake() {
ctx.fillStyle = "green";
for (var i = 0; i < snake.length; i++) {
ctx.fillRect(snake[i].x * 10, snake[i].y * 10, 10, 10);
}
}
function drawFood() {
ctx.fillStyle = "red";
ctx.fillRect(food.x * 10, food.y * 10, 10, 10);
}
function generateFood() {
food.x = Math.floor(Math.random() * 40);
food.y = Math.floor(Math.random() * 40);
}
function moveSnake() {
var head = {x: snake[0].x, y: snake[0].y};
if (direction == "right") {
head.x++;
} else if (direction == "left") {
head.x--;
} else if (direction == "up") {
head.y--;
} else if (direction == "down") {
head.y++;
}
snake.unshift(head);
if (head.x == food.x && head.y == food.y) {
score++;
generateFood();
} else {
snake.pop();
}
}
function checkCollision() {
var head = snake[0];
if (head.x < 0 || head.x >= 40 || head.y < 0 || head.y >= 40) {
return true;
}
for (var i = 1; i < snake.length; i++) {
if (head.x == snake[i].x && head.y == snake[i].y) {
return true;
}
}
return false;
}
function update() {
moveSnake();
if (checkCollision()) {
clearInterval(interval);
alert("游戏结束!得分:" + score);
}
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawSnake();
drawFood();
}
generateFood();
var interval = setInterval(update, 100);
document.addEventListener("keydown", function(event) {
if (event.keyCode == 37 && direction != "right") {
direction = "left";
} else if (event.keyCode == 38 && direction != "down") {
direction = "up";
} else if (event.keyCode == 39 && direction != "left") {
direction = "right";
} else if (event.keyCode == 40 && direction != "up") {
direction = "down";
}
});
```
这个小游戏使用 canvas 元素绘制,通过 JavaScript 控制蛇的移动和食物的生成,使用键盘事件监听器控制蛇的方向。
阅读全文
相关推荐
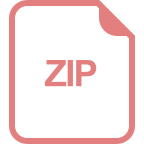




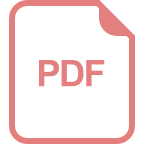
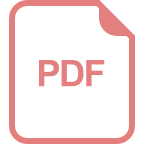
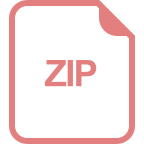
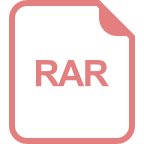






