用JavaScript写一个HTML5贪吃蛇小游戏
时间: 2023-09-03 13:07:23 浏览: 39
好的,以下是一个简单的HTML5贪吃蛇小游戏的JavaScript代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>贪吃蛇小游戏</title>
<style>
#game-board {
width: 500px;
height: 500px;
border: 1px solid black;
margin: auto;
}
</style>
</head>
<body>
<div id="game-board"></div>
<script>
// 获取游戏板元素
const gameBoard = document.getElementById('game-board');
// 定义游戏板参数
const boardWidth = 20;
const boardHeight = 20;
const squareSize = 25;
// 定义蛇参数
let snake = [{x: 10, y: 10}];
let direction = 'right';
// 定义食物参数
let food = {x: 5, y: 5};
// 绘制游戏板
function drawBoard() {
let squares = '';
for (let y = 0; y < boardHeight; y++) {
for (let x = 0; x < boardWidth; x++) {
squares += `<div class="square" style="width:${squareSize}px;height:${squareSize}px;"></div>`;
}
}
gameBoard.innerHTML = squares;
}
// 绘制蛇和食物
function drawSnakeAndFood() {
const squares = gameBoard.querySelectorAll('.square');
// 绘制蛇
snake.forEach((segment) => {
const snakeSquare = squares[segment.x + segment.y * boardWidth];
snakeSquare.classList.add('snake');
});
// 绘制食物
const foodSquare = squares[food.x + food.y * boardWidth];
foodSquare.classList.add('food');
}
// 移动蛇
function moveSnake() {
// 计算新蛇头位置
const currentHead = snake[snake.length - 1];
let newHead;
switch (direction) {
case 'right':
newHead = {x: currentHead.x + 1, y: currentHead.y};
break;
case 'left':
newHead = {x: currentHead.x - 1, y: currentHead.y};
break;
case 'up':
newHead = {x: currentHead.x, y: currentHead.y - 1};
break;
case 'down':
newHead = {x: currentHead.x, y: currentHead.y + 1};
break;
}
// 检查是否撞墙或撞到自己
if (newHead.x < 0 || newHead.x >= boardWidth || newHead.y < 0 || newHead.y >= boardHeight || snake.some((segment) => segment.x === newHead.x && segment.y === newHead.y)) {
clearInterval(gameLoop);
alert('游戏结束!');
return;
}
// 检查是否吃到食物
if (newHead.x === food.x && newHead.y === food.y) {
food = {x: Math.floor(Math.random() * boardWidth), y: Math.floor(Math.random() * boardHeight)};
} else {
snake.shift(); // 移除蛇尾
}
// 添加新蛇头
snake.push(newHead);
}
// 更新游戏状态
function updateGame() {
moveSnake();
drawSnakeAndFood();
}
// 处理键盘事件
function handleKeyDown(event) {
switch (event.keyCode) {
case 37: // 左箭头
if (direction !== 'right') {
direction = 'left';
}
break;
case 38: // 上箭头
if (direction !== 'down') {
direction = 'up';
}
break;
case 39: // 右箭头
if (direction !== 'left') {
direction = 'right';
}
break;
case 40: // 下箭头
if (direction !== 'up') {
direction = 'down';
}
break;
}
}
// 初始化游戏
function initGame() {
drawBoard();
drawSnakeAndFood();
document.addEventListener('keydown', handleKeyDown);
gameLoop = setInterval(updateGame, 150);
}
// 开始游戏
initGame();
</script>
</body>
</html>
```
在页面上运行这个代码后,你可以通过方向键来控制蛇的移动,尝试吃到食物并躲避墙壁和自己的身体。
相关推荐
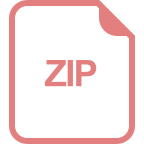
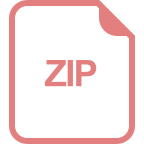
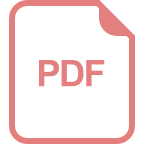














