写一个提取execl某一列所有字母和数字的代码
时间: 2024-02-20 22:57:36 浏览: 21
可以使用Python的pandas库来实现提取Excel某一列所有字母和数字的功能。示例代码如下:
```python
import pandas as pd
# 读取Excel文件
df = pd.read_excel('your_excel_file.xlsx')
# 提取某一列的所有值
column_values = df['your_column_name'].values.tolist()
# 提取所有字母和数字
alphanumeric_values = []
for value in column_values:
if isinstance(value, str):
alphanumeric_values.extend([char for char in value if char.isalnum()])
# 输出结果
print(alphanumeric_values)
```
其中,`your_excel_file.xlsx`是你要读取的Excel文件名,`your_column_name`是你要提取的列名。这段代码会将该列中所有的字母和数字提取出来,并存储在`alphanumeric_values`列表中。
相关问题
写一个提取execl某一列提取字母开头的数字结尾的代码
可以使用Python的pandas库来实现提取Excel某一列中以字母开头、数字结尾的数据。示例代码如下:
```python
import pandas as pd
import re
# 读取Excel文件
df = pd.read_excel('your_excel_file.xlsx')
# 提取某一列的所有值
column_values = df['your_column_name'].values.tolist()
# 提取以字母开头、数字结尾的数据
pattern = re.compile(r'[A-Za-z]\d+$')
result = []
for value in column_values:
if isinstance(value, str):
match = pattern.search(value)
if match:
result.append(match.group())
# 输出结果
print(result)
```
其中,`your_excel_file.xlsx`是你要读取的Excel文件名,`your_column_name`是你要提取的列名。这段代码会将该列中所有以字母开头、数字结尾的数据提取出来,并存储在`result`列表中。使用正则表达式`[A-Za-z]\d+$`来匹配以字母开头、数字结尾的数据。
用java写一个代码提取execl表某一列字母开头数字结尾的字符串
可以使用Apache POI库来读取Excel表格,并使用正则表达式匹配某一列的单元格内容。以下是一个例子代码:
```java
import java.io.FileInputStream;
import java.io.IOException;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelParser {
public static void main(String[] args) throws IOException {
String fileName = "example.xlsx"; // Excel文件名
int columnIndex = 2; // 要匹配的列的索引,从0开始计数
String pattern = "^\\w+\\d+$"; // 匹配模式,字母开头数字结尾的字符串
FileInputStream file = new FileInputStream(fileName);
Workbook workbook = new XSSFWorkbook(file);
Sheet sheet = workbook.getSheetAt(0); // 获取第一个工作表
Pattern regex = Pattern.compile(pattern);
for (Row row : sheet) {
Cell cell = row.getCell(columnIndex);
if (cell != null && cell.getCellType() == Cell.CELL_TYPE_STRING) {
String value = cell.getStringCellValue();
Matcher matcher = regex.matcher(value);
if (matcher.matches()) {
System.out.println(value);
}
}
}
workbook.close();
file.close();
}
}
```
这个例子会读取Excel文件中第一个工作表的每一行,提取指定列的单元格内容,并使用正则表达式匹配符合要求的字符串。如果找到符合要求的字符串,就会输出它们的值。你可以根据需要修改文件名、列索引和匹配模式等参数。
相关推荐
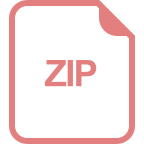
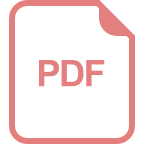
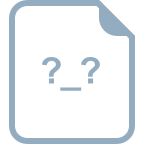












