使用Java编程和springboot和mybatis框架,连接mysql数据库,给出具体程序,包含Controller层、Service层、Service实现类、Dao层以及Mapper.xml文件,统计本周第一天 本月第一天 本年第一天起到今天为止的中奖趋势接口 并对接口进行redis缓存处理 过期时间10分钟
时间: 2024-03-26 08:38:21 浏览: 11
好的,以下是具体程序:
首先,需要在pom.xml中添加相关依赖:
```
<!-- SpringBoot -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.4.4</version>
</dependency>
<!-- Mybatis -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.4</version>
</dependency>
<!-- MySQL Connector -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.23</version>
</dependency>
<!-- Redis -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
<version>2.4.4</version>
</dependency>
```
接下来,创建数据库表,并在`application.properties`文件中配置数据库连接信息:
```
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/test?useSSL=false&serverTimezone=UTC&useUnicode=true&characterEncoding=utf-8
spring.datasource.username=root
spring.datasource.password=123456
mybatis.type-aliases-package=com.example.demo.entity
mybatis.mapper-locations=classpath:mapper/*.xml
```
然后,创建实体类`Lottery`:
```
public class Lottery {
private Integer id;
private String name;
private String date;
private Integer num;
// getter and setter
}
```
接着,创建`LotteryMapper.xml`文件,定义对应的SQL语句:
```
<mapper namespace="com.example.demo.mapper.LotteryMapper">
<resultMap id="LotteryMap" type="com.example.demo.entity.Lottery">
<id property="id" column="id" />
<result property="name" column="name" />
<result property="date" column="date" />
<result property="num" column="num" />
</resultMap>
<select id="getLotteryListByDate" resultMap="LotteryMap">
SELECT id, name, date, num
FROM lottery
WHERE date BETWEEN #{startDate} AND #{endDate}
ORDER BY date ASC
</select>
</mapper>
```
然后,创建`LotteryMapper`接口:
```
public interface LotteryMapper {
List<Lottery> getLotteryListByDate(@Param("startDate") String startDate, @Param("endDate") String endDate);
}
```
接着,创建`LotteryService`接口和实现类`LotteryServiceImpl`:
```
public interface LotteryService {
List<Lottery> getLotteryListByDate(String startDate, String endDate);
}
@Service
public class LotteryServiceImpl implements LotteryService {
@Autowired
private LotteryMapper mapper;
@Override
@Cacheable(value = "lottery", key = "#startDate + '-' + #endDate")
public List<Lottery> getLotteryListByDate(String startDate, String endDate) {
return mapper.getLotteryListByDate(startDate, endDate);
}
}
```
注意到`getLotteryListByDate`方法上使用了`@Cacheable`注解,表示对该方法进行缓存处理,并设置了缓存的名称为`lottery`,缓存的key为`startDate + '-' + endDate`,即根据日期作为缓存的key。
最后,创建`LotteryController`,定义对外接口:
```
@RestController
@RequestMapping("/lottery")
public class LotteryController {
@Autowired
private LotteryService service;
@GetMapping("/trend")
public List<Lottery> getTrend() {
LocalDate now = LocalDate.now();
String weekStart = now.with(DayOfWeek.MONDAY).toString();
String monthStart = now.withDayOfMonth(1).toString();
String yearStart = now.withDayOfYear(1).toString();
List<Lottery> weekTrend = service.getLotteryListByDate(weekStart, now.toString());
List<Lottery> monthTrend = service.getLotteryListByDate(monthStart, now.toString());
List<Lottery> yearTrend = service.getLotteryListByDate(yearStart, now.toString());
List<Lottery> trend = new ArrayList<>();
trend.addAll(weekTrend);
trend.addAll(monthTrend);
trend.addAll(yearTrend);
return trend;
}
}
```
其中,`getTrend`方法获取本周、本月、本年的数据,并返回合并后的结果。
至此,程序已经完成,可以启动SpringBoot应用进行测试。同时,缓存的过期时间默认为10分钟,可以在`application.properties`文件中进行设置:
```
spring.cache.redis.time-to-live=600000
```
相关推荐
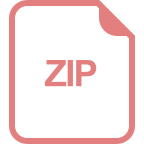
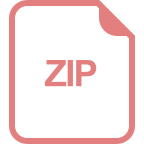
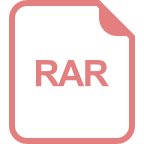








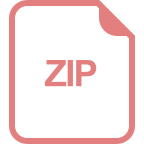
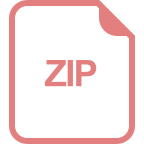
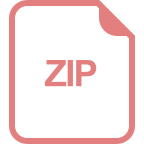
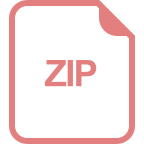
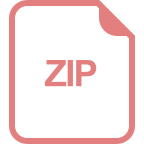
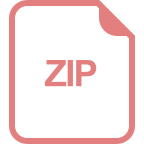
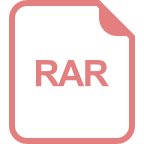