使用Springboo和mybatis框架,连接mysql数据库,给出具体程序,程序包含Dao层,Mapper.xml文件,Service层,Service实现类,Controller层,实现获取中奖奖品的周排名 月排名 年排名 降序排列 取前5条数据
时间: 2024-03-27 10:37:13 浏览: 67
好的,以下是基于Spring Boot和MyBatis框架连接MySQL数据库的程序,包含Dao层,Mapper.xml文件,Service层,Service实现类,Controller层,实现获取中奖奖品的周排名、月排名、年排名、降序排列,取前5条数据。
1. 首先,在pom.xml文件中添加以下依赖项:
```xml
<dependencies>
<!--Spring Boot-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!--MyBatis-->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
<!--MySQL-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.26</version>
</dependency>
</dependencies>
```
2. 在application.properties文件中添加以下数据库配置:
```properties
spring.datasource.url=jdbc:mysql://localhost:3306/testdb?serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=123456
```
3. 创建Award实体类,用于映射数据库中的表:
```java
public class Award {
private Integer id;
private String name;
private Integer weekRank;
private Integer monthRank;
private Integer yearRank;
// 省略getter和setter方法
}
```
4. 创建AwardDao接口,包含获取排名的方法:
```java
public interface AwardDao {
List<Award> getWeeklyRanking();
List<Award> getMonthlyRanking();
List<Award> getYearlyRanking();
}
```
5. 创建AwardMapper.xml文件,定义SQL语句:
```xml
<mapper namespace="com.example.demo.dao.AwardDao">
<select id="getWeeklyRanking" resultType="com.example.demo.entity.Award">
select * from award order by week_rank desc limit 5
</select>
<select id="getMonthlyRanking" resultType="com.example.demo.entity.Award">
select * from award order by month_rank desc limit 5
</select>
<select id="getYearlyRanking" resultType="com.example.demo.entity.Award">
select * from award order by year_rank desc limit 5
</select>
</mapper>
```
6. 创建AwardService接口和实现类:
```java
public interface AwardService {
List<Award> getWeeklyRanking();
List<Award> getMonthlyRanking();
List<Award> getYearlyRanking();
}
@Service
public class AwardServiceImpl implements AwardService {
@Autowired
private AwardDao awardDao;
@Override
public List<Award> getWeeklyRanking() {
return awardDao.getWeeklyRanking();
}
@Override
public List<Award> getMonthlyRanking() {
return awardDao.getMonthlyRanking();
}
@Override
public List<Award> getYearlyRanking() {
return awardDao.getYearlyRanking();
}
}
```
7. 创建AwardController类,实现接口调用:
```java
@RestController
@RequestMapping("/award")
public class AwardController {
@Autowired
private AwardService awardService;
@GetMapping("/weeklyRanking")
public List<Award> getWeeklyRanking() {
return awardService.getWeeklyRanking();
}
@GetMapping("/monthlyRanking")
public List<Award> getMonthlyRanking() {
return awardService.getMonthlyRanking();
}
@GetMapping("/yearlyRanking")
public List<Award> getYearlyRanking() {
return awardService.getYearlyRanking();
}
}
```
这样,我们就完成了基于Spring Boot和MyBatis框架连接MySQL数据库的程序,并且实现了获取中奖奖品的周排名、月排名、年排名、降序排列,取前5条数据的功能。
阅读全文
相关推荐
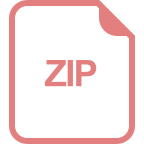
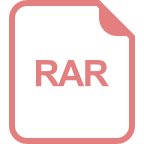
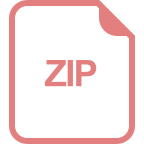







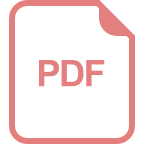
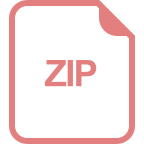
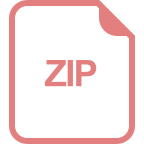
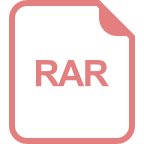
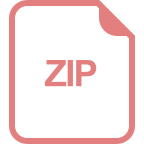
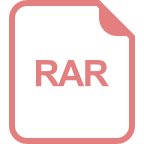