python文件如何读写字典和列表
时间: 2024-12-15 08:16:45 浏览: 3
在Python中,文件操作通常涉及到字典和列表这样的数据结构。你可以使用内置的`json`模块或者简单的文本文件来进行读写。
**读取字典(JSON文件):**
```python
import json
# 写入字典到文件
data = {'name': 'John', 'age': 30}
with open('dict_file.json', 'w') as f:
json.dump(data, f)
# 从文件读取字典
with open('dict_file.json', 'r') as f:
loaded_data = json.load(f)
print(loaded_data) # 输出:{'name': 'John', 'age': 30}
```
这里使用了`json.dump()`函数将字典序列化为JSON格式写入文件,然后`json.load()`函数用于反序列化恢复成字典。
**读写列表(CSV文件为例,假设列名已知):**
```python
import csv
# 写入列表到CSV文件(逗号分隔)
data = ['Name', 'Age']
with open('list_file.csv', 'w', newline='') as csvfile:
writer = csv.writer(csvfile)
writer.writerow(data)
# 从CSV文件读取列表
with open('list_file.csv', 'r') as csvfile:
reader = csv.reader(csvfile)
next(reader) # 跳过标题行
list_data = [row for row in reader]
print(list_data) # 输出:[['Name'], ['Age']]
```
这里使用`csv.writer`和`csv.reader`处理CSV文件。对于列表,我们通常会跳过第一行(标题行),并将后续行读取到一个新的列表中。
阅读全文
相关推荐
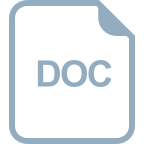
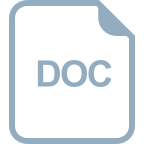
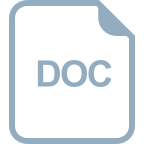
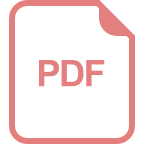
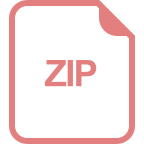
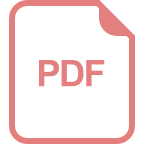
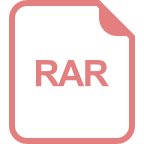
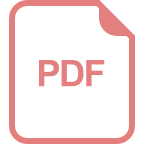
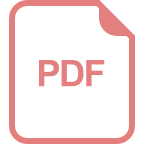
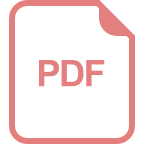
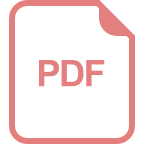
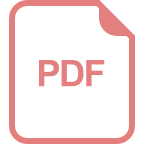
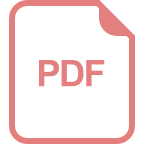
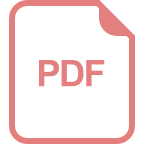
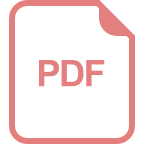
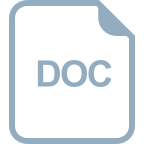
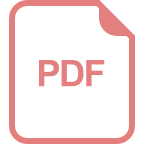
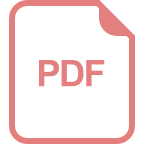
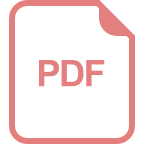