python爬取京东数据并分析
时间: 2023-06-26 18:04:55 浏览: 364
好的,下面是一个简单的爬取京东商品信息并分析的 Python 代码示例:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
# 设置请求头
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 爬取京东商品列表页面,获取商品链接
def get_links(url):
try:
r = requests.get(url, headers=headers)
soup = BeautifulSoup(r.text, 'html.parser')
links = []
for item in soup.select('.gl-item .p-name a'):
links.append(item.get('href'))
return links
except:
print('Error: Failed to get links')
# 爬取京东商品详情页面,获取商品信息
def get_info(url):
try:
r = requests.get(url, headers=headers)
soup = BeautifulSoup(r.text, 'html.parser')
name = soup.select('.sku-name')[0].text.strip()
price = soup.select('.price .price-text')[0].text.strip()
comment_count = soup.select('#comment-count')[0].text.strip()
shop_name = soup.select('.J-summary-shop-name')[0].text.strip()
return {'name': name, 'price': price, 'comment_count': comment_count, 'shop_name': shop_name}
except:
print('Error: Failed to get info')
# 主程序
if __name__ == '__main__':
keyword = '手机' # 搜索关键词
url = 'https://search.jd.com/Search?keyword=' + keyword
links = get_links(url)
data = []
for link in links:
if 'item.jd.com' in link:
info = get_info(link)
data.append(info)
df = pd.DataFrame(data)
df.to_csv('jd.csv', encoding='utf-8-sig')
print('Done!')
```
这个示例程序可以爬取京东搜索页面中包含指定关键词的商品信息,并将商品名称、价格、评论数和店铺名称等信息导出为 CSV 文件。你可以在获取数据之后使用 pandas 库进行分析,例如:
```python
import pandas as pd
# 加载 CSV 文件
df = pd.read_csv('jd.csv', encoding='utf-8-sig')
# 统计价格分布情况
price_counts = df['price'].value_counts()
print(price_counts)
# 统计评论数分布情况
comment_counts = df['comment_count'].value_counts()
print(comment_counts)
# 统计店铺数量
shop_count = len(df['shop_name'].unique())
print(shop_count)
```
这只是一个简单的示例,你可以根据自己的需求进行更多的数据分析和处理。注意,爬取网站数据涉及到法律和道德等方面的问题,请遵守相关规定和行为准则。
阅读全文
相关推荐
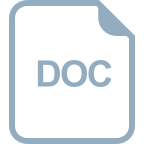
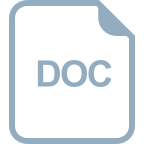
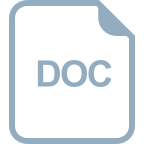
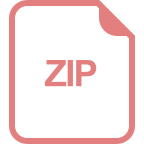







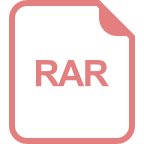





