最后的部分请用c语言画图替代python画图
时间: 2023-07-24 09:32:41 浏览: 97
好的,如果要使用C语言绘制图表,可以使用GNU plot库。需要安装GNU plot库后,在C语言中使用`popen`函数来调用GNU plot命令。以下是一个简单的例子:
```
#include <stdio.h>
int main() {
FILE *gnuplot = popen("gnuplot", "w");
fprintf(gnuplot, "plot sin(x)\n");
fflush(gnuplot);
getchar();
pclose(gnuplot);
return 0;
}
```
这个例子会绘制正弦函数的图像,并在按下回车键后关闭GNU plot窗口。
我们可以根据上面Python的代码,将每个流接收到的总字节和时间输出到文件中,然后使用GNU plot绘制图表。以下是示例代码:
```
#include <stdio.h>
#include <stdlib.h>
struct PacketInfo {
double timestamp;
int flowID;
int size;
};
int main() {
// 读取数据包信息
FILE *fp = fopen("packet_info.txt", "r");
if (fp == NULL) {
perror("fopen");
exit(1);
}
struct PacketInfo packet;
int flow_count = 0;
int *flow_bytes = NULL;
double *flow_time = NULL;
while (fscanf(fp, "%lf %d %d\n", &packet.timestamp, &packet.flowID, &packet.size) == 3) {
if (packet.flowID >= flow_count) {
flow_count = packet.flowID + 1;
flow_bytes = realloc(flow_bytes, flow_count * sizeof(int));
flow_time = realloc(flow_time, flow_count * sizeof(double));
for (int i = 0; i < flow_count; i++) {
flow_bytes[i] = 0;
flow_time[i] = -1;
}
}
if (flow_time[packet.flowID] < 0) {
flow_time[packet.flowID] = packet.timestamp;
}
flow_bytes[packet.flowID] += packet.size;
}
fclose(fp);
// 输出到文件
fp = fopen("plot_data.txt", "w");
if (fp == NULL) {
perror("fopen");
exit(1);
}
for (int i = 0; i < flow_count; i++) {
if (flow_time[i] < 0) {
continue;
}
fprintf(fp, "# Flow %d\n", i);
int bytes = 0;
for (double t = 0; t < flow_time[i] + 10; t += 0.1) {
while (bytes < flow_bytes[i] && flow_time[0] + t >= flow_time[i] + (double)bytes / flow_bytes[i]) {
bytes++;
}
fprintf(fp, "%lf %d\n", t, bytes);
}
fprintf(fp, "\n");
}
fclose(fp);
// 绘制图表
FILE *gnuplot = popen("gnuplot", "w");
fprintf(gnuplot, "set title 'Bytes Received'\n");
fprintf(gnuplot, "set xlabel 'Time (s)'\n");
fprintf(gnuplot, "set ylabel 'Total Bytes Received'\n");
fprintf(gnuplot, "plot 'plot_data.txt' every ::1 using 1:2 with lines title 'Flow 0'");
for (int i = 1; i < flow_count; i++) {
fprintf(gnuplot, ", '' every ::%d using 1:2 with lines title 'Flow %d'", i * 2 - 1, i);
}
fprintf(gnuplot, "\n");
fflush(gnuplot);
getchar();
pclose(gnuplot);
// 释放内存
free(flow_bytes);
free(flow_time);
return 0;
}
```
这个例子会读取之前输出到文件中的数据包信息,然后输出到另一个文件中,并使用GNU plot绘制图表。需要注意的是,为了使每个流的图像不重叠,我们在每个流的数据之间添加了一个空行,这样GNU plot会自动将它们分开。另外,由于GNU plot不支持在图例中使用变量,我们需要手动为每个流设置一个标题。
阅读全文
相关推荐
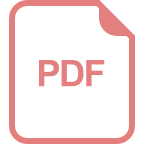
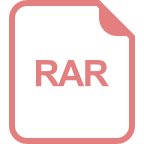
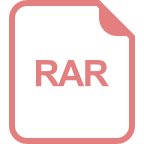
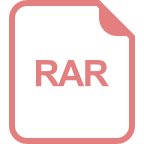
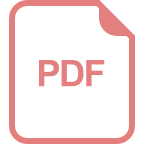
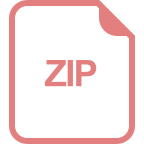
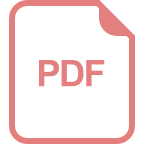
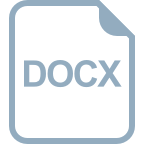
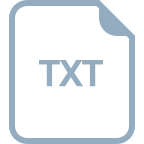
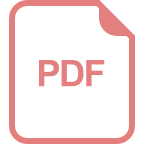
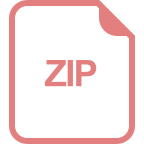
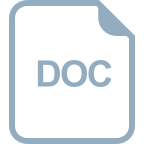
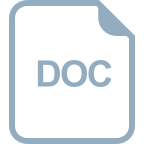
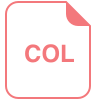
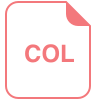
