用c++用友元函数重载运算符运算符“+”和“-”将两个二维数组相加和相减。
时间: 2023-11-13 21:05:09 浏览: 53
好的,我来解答你的问题。
首先,我们需要定义一个二维数组类,然后重载运算符“+”和“-”。在重载运算符时,我们需要使用友元函数来访问私有成员变量。
以下是一个示例代码:
```c++
#include <iostream>
using namespace std;
class Array2D {
private:
int** data;
int row, col;
public:
Array2D(int r, int c) {
row = r;
col = c;
data = new int*[row];
for (int i = 0; i < row; i++) {
data[i] = new int[col];
for (int j = 0; j < col; j++) {
data[i][j] = 0;
}
}
}
~Array2D() {
for (int i = 0; i < row; i++) {
delete[] data[i];
}
delete[] data;
}
int& operator()(int i, int j) {
return data[i][j];
}
friend Array2D operator+(const Array2D& a, const Array2D& b) {
if (a.row != b.row || a.col != b.col) {
throw "Error: Array2D size mismatch.";
}
Array2D result(a.row, a.col);
for (int i = 0; i < a.row; i++) {
for (int j = 0; j < a.col; j++) {
result(i, j) = a.data[i][j] + b.data[i][j];
}
}
return result;
}
friend Array2D operator-(const Array2D& a, const Array2D& b) {
if (a.row != b.row || a.col != b.col) {
throw "Error: Array2D size mismatch.";
}
Array2D result(a.row, a.col);
for (int i = 0; i < a.row; i++) {
for (int j = 0; j < a.col; j++) {
result(i, j) = a.data[i][j] - b.data[i][j];
}
}
return result;
}
void print() {
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
cout << data[i][j] << " ";
}
cout << endl;
}
}
};
int main() {
Array2D a(2, 2);
Array2D b(2, 2);
a(0, 0) = 1;
a(0, 1) = 2;
a(1, 0) = 3;
a(1, 1) = 4;
b(0, 0) = 5;
b(0, 1) = 6;
b(1, 0) = 7;
b(1, 1) = 8;
cout << "a:" << endl;
a.print();
cout << "b:" << endl;
b.print();
cout << "a+b:" << endl;
Array2D c = a + b;
c.print();
cout << "a-b:" << endl;
Array2D d = a - b;
d.print();
return 0;
}
```
在上面的示例中,我们首先定义了一个二维数组类`Array2D`,其中包含了私有成员变量`data`、`row`和`col`,以及公有成员函数`operator()`和`print`。`operator()`函数用于访问二维数组中的元素,而`print`函数用于打印二维数组中的所有元素。
接下来,我们重载了运算符“+”和“-”,并使用了友元函数来访问`Array2D`类的私有成员变量。在重载运算符时,我们首先检查两个数组的大小是否相同,如果不同则抛出异常。然后,我们创建一个新的`Array2D`对象,将两个数组对应位置的元素相加或相减,并将结果保存到新的数组中,最后返回该数组。
在主函数中,我们创建了两个二维数组`a`和`b`,并对其进行了初始化。然后,我们分别使用重载的运算符“+”和“-”将两个数组相加和相减,并将结果保存到新的数组中,最后打印结果。
希望这个示例代码可以帮助你理解如何使用C++实现友元函数重载运算符。
相关推荐
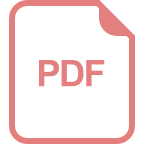
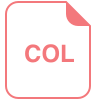
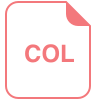
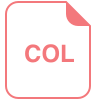
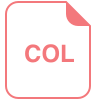










