python pdf批注
时间: 2023-10-04 20:05:07 浏览: 189
您可以使用Python中的PyPDF2库来批注PDF文件。以下是一个简单的示例代码来实现这个功能:
```python
import PyPDF2
def annotate_pdf(input_path, output_path, page_number, x, y, text):
# 打开PDF文件
with open(input_path, 'rb') as file:
# 创建一个PDF阅读器对象
reader = PyPDF2.PdfReader(file)
# 创建一个PDF写入器对象
writer = PyPDF2.PdfWriter()
# 循环遍历每一页
for page in reader.pages:
# 复制原始页面
new_page = page
# 如果是指定的页面,则添加注释
if page_number == reader.pages.index(page):
annotation = PyPDF2.pdf.PageAnnotation()
annotation.Text = text
annotation.Rect = [x, y, x + 100, y + 20]
new_page.Annots.append(annotation)
# 将新页面添加到写入器中
writer.add_page(new_page)
# 将写入器中的内容写入输出文件
with open(output_path, 'wb') as output_file:
writer.write(output_file)
# 示例用法
input_path = 'input.pdf'
output_path = 'output.pdf'
page_number = 0 # 第一页的索引为0
x = 100 # 注释左上角的x坐标
y = 100 # 注释左上角的y坐标
text = '这是一个批注' # 注释文本
annotate_pdf(input_path, output_path, page_number, x, y, text)
```
在上述代码中,您需要指定输入PDF文件的路径 (`input_path`)、输出PDF文件的路径 (`output_path`)、要批注的页面索引 (`page_number`)、注释位置的左上角坐标 (`x` 和 `y`),以及注释的文本 (`text`)。然后,代码会打开输入PDF文件,并遍历每一页。如果页码与指定的页面索引相匹配,则在该页面上添加一个注释。最后,代码将写入器中的内容写入输出文件。
请注意,这个示例只是一个简单的演示,如果您需要更复杂的批注功能,可能需要使用更高级的PDF处理库,如ReportLab或PyMuPDF。
阅读全文
相关推荐


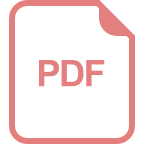
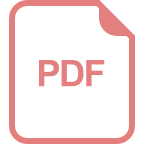
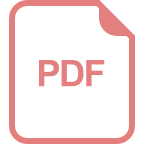
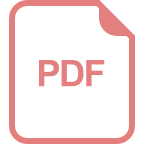
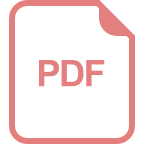
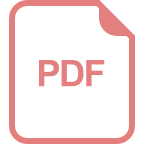
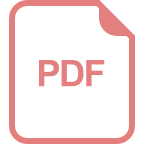
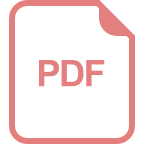
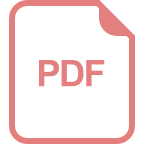
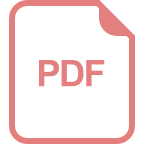
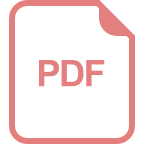
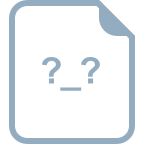
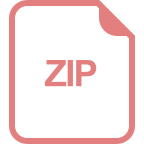
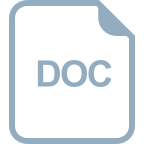