点云ptd格式读写 c++自实现其过程
时间: 2023-08-18 21:06:07 浏览: 192
点云PTD格式(Point Data)是一种简单的文本格式,用于存储点云数据。它由一系列的点组成,每个点由三个浮点数表示其在三维空间中的位置。在这个格式中,每个点占据一行,每个点的坐标由空格或制表符分隔。下面是一个简单的PTD文件的例子:
```
0.1 0.2 0.3
0.4 0.5 0.6
0.7 0.8 0.9
```
下面是C++实现读取和写入PTD格式文件的示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
struct Point {
float x, y, z;
};
void read_ptd(const std::string& filename, std::vector<Point>& points) {
std::ifstream infile(filename);
if (!infile.is_open()) {
std::cerr << "Error: Cannot open file " << filename << std::endl;
return;
}
points.clear();
std::string line;
while (std::getline(infile, line)) {
Point p;
if (sscanf(line.c_str(), "%f %f %f", &p.x, &p.y, &p.z) == 3)
points.push_back(p);
}
}
void write_ptd(const std::string& filename, const std::vector<Point>& points) {
std::ofstream outfile(filename);
if (!outfile.is_open()) {
std::cerr << "Error: Cannot open file " << filename << std::endl;
return;
}
for (const auto& p : points)
outfile << p.x << " " << p.y << " " << p.z << std::endl;
}
int main() {
std::vector<Point> points;
read_ptd("input.ptd", points);
// do something with the points
write_ptd("output.ptd", points);
return 0;
}
```
这个程序中,我们定义了一个名为`Point`的结构体,用来表示每个点的坐标。`read_ptd`函数接受一个文件名和一个点向量,它打开指定的文件并按行读取文件内容。对于每一行,它将读取三个浮点数,构造一个`Point`结构体,并将其添加到点向量中。`write_ptd`函数接受一个文件名和一个点向量,它打开指定的文件并按行写入所有点的坐标。
在主函数中,我们可以调用`read_ptd`函数读取一个PTD格式的文件,然后对点进行处理。最后,我们可以将处理后的点写入到一个新的PTD文件中,通过调用`write_ptd`函数。
这是一个简单的实现,你可以根据需要进行修改和扩展。
阅读全文
相关推荐
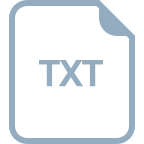
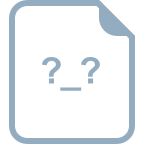
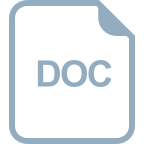

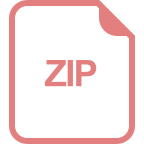
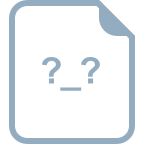
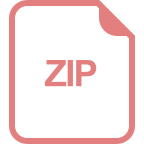
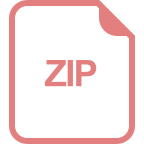
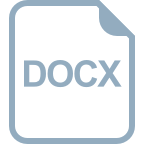
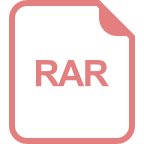




