编写函数,将输入字符串的前3个字符移到最后。 函数接口定义: void Shift( char s[] ); 其中char s[]是用户传入的字符串,题目保证其长度不小于3;函数Shift须将按照要求变换后的字符串仍然存在s[]里,C语言具体实现
时间: 2024-02-22 17:56:25 浏览: 61
下面是函数Shift的C语言实现:
```c
void Shift(char s[]) {
char temp = s[0];
for (int i = 0; i < strlen(s) - 1; i++) {
s[i] = s[i + 1];
}
s[strlen(s) - 1] = temp;
temp = s[0];
for (int i = 0; i < strlen(s) - 1; i++) {
s[i] = s[i + 1];
}
s[strlen(s) - 1] = temp;
temp = s[0];
for (int i = 0; i < strlen(s) - 1; i++) {
s[i] = s[i + 1];
}
s[strlen(s) - 1] = temp;
}
```
函数中,我们首先将第一个字符存入临时变量temp中,然后将字符串中的每个字符向左移动一位,最后将temp存入字符串的最后一位,这样就完成了一次移位。我们将这个操作重复执行3次即可将前3个字符移到最后。注意,需要包含头文件`string.h`以使用strlen函数。
相关问题
题要求编写函数,将输入字符串的前3个字符移到最后。 函数接口定义: void Shift( char s[] ); 其中char s[]是用户传入的字符串,题目保证其长度不小于3;函数Shift须将按照要求变换后的字符串仍然存在s[]里。
void Shift(char s[]) {
char temp = s[0];
for (int i = 0; i < strlen(s) - 1; i++) {
s[i] = s[i + 1];
}
s[strlen(s) - 1] = temp;
temp = s[0];
for (int i = 0; i < strlen(s) - 1; i++) {
s[i] = s[i + 1];
}
s[strlen(s) - 1] = temp;
temp = s[0];
for (int i = 0; i < strlen(s) - 1; i++) {
s[i] = s[i + 1];
}
s[strlen(s) - 1] = temp;
}
本题要求编写函数,将输入字符串的前3个字符移到最后。 函数接口定义: void shift( char s[] ); 其中char s[]是用户传入的字符串,题目保证其长度不小于3;函数shift须将按照要求变换后的字符串仍然存在s[]里。
好的,以下是函数shift的中文实现:
```c++
void shift(char s[]) {
// 将前3个字符存储到一个临时数组中
char temp[3];
for (int i = 0; i < 3; i++) {
temp[i] = s[i];
}
// 将剩余的字符往前移动3个位置
for (int i = 0; s[i + 3] != '\0'; i++) {
s[i] = s[i + 3];
}
// 将临时数组中存储的字符放到末尾3个位置上
for (int i = 0; i < 3; i++) {
s[strlen(s) - 3 + i] = temp[i];
}
}
```
这个函数首先将输入字符串的前3个字符存储到一个临时数组中,然后将剩余的字符往前移动3个位置,最后将临时数组中存储的字符放到末尾3个位置上,从而实现了将输入字符串的前3个字符移到最后的功能。
阅读全文
相关推荐
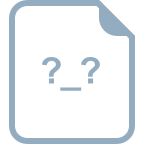
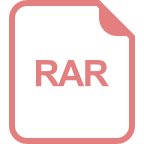














