本题要求编写函数,将输入字符串的前3个字符移到最后。完善并优化以下代码#include <stdio.h> #include <string.h> #define MAXS 10 void Shift( char s[] ); void GetString( char s[] ); /* 实现细节在此不表 */ int main() { char s[MAXS]; GetString(s); Shift(s); printf("%s\n", s); return 0; } void GetString( char s[]) { gets(s); } void Shift(char s[]) { char ch[30]; ch = strcpy(s,s+2); }
时间: 2024-03-07 22:50:16 浏览: 19
代码存在以下问题:
1. 使用了不安全的 gets 函数,应该改用 fgets 函数。
2. Shift 函数中,使用了一个字符数组 ch,但是并没有使用到,而且将 s 的前三个字符移动到 s 的末尾应该使用循环实现。
以下是修改后的代码:
```c
#include <stdio.h>
#include <string.h>
#define MAXS 10
void Shift(char s[]);
int main() {
char s[MAXS];
fgets(s, MAXS, stdin);
Shift(s);
printf("%s\n", s);
return 0;
}
void Shift(char s[]) {
int len = strlen(s);
char tmp;
for (int i = 0; i < 3; i++) {
tmp = s[i];
for (int j = i; j < len - 1; j += 3) {
s[j] = s[j + 3];
}
s[len - 3 + i] = tmp;
}
}
```
修改后的 Shift 函数中,首先获取字符串的长度 len,然后使用两层循环,将前三个字符依次移动到字符串的末尾。其中,外层循环控制移动的字符个数,内层循环控制字符的移动位置。
相关问题
解释这三行#include <stdlib.h> #include <string.h> #include <stdio.h>
这三行代码是C语言中的预处理指令,用于引入标准库中的函数和定义。具体解释如下:
1. `#include <stdlib.h>`:引入stdlib.h头文件,该头文件包含了一些常用的函数,如内存分配、类型转换、随机数生成等函数。
2. `#include <string.h>`:引入string.h头文件,该头文件包含了一些字符串处理函数,如字符串复制、字符串比较、字符串连接等函数。
3. `#include <stdio.h>`:引入stdio.h头文件,该头文件包含了一些输入输出函数,如文件操作、标准输入输出等函数。
这些头文件中的函数可以在程序中直接调用,以实现相应的功能。例如,可以使用stdlib.h中的函数来进行内存分配和释放,使用string.h中的函数来处理字符串,使用stdio.h中的函数来进行文件操作和标准输入输出。
对以下代码纠正#include<stdio.h> #include<stdlib.h> #include<string.h> #include<math.h> #define MaxSize 100 #define ElemType int #define Status int
这段代码是一个C语言的头文件引用部分,它包含了一些常用的标准库和宏定义。具体解释如下:
1. `#include<stdio.h>`:引用了标准输入输出库,可以使用printf和scanf等函数。
2. `#include<stdlib.h>`:引用了标准库,可以使用malloc和free等函数进行内存管理。
3. `#include<string.h>`:引用了字符串处理库,可以使用strcpy和strlen等函数进行字符串操作。
4. `#include<math.h>`:引用了数学库,可以使用数学函数如sin和cos等。
5. `#define MaxSize 100`:定义了一个宏常量MaxSize,表示数组的最大长度为100。
6. `#define ElemType int`:定义了一个宏常量ElemType,表示数组元素的类型为int。
7. `#define Status int`:定义了一个宏常量Status,表示函数返回的状态类型为int。
这些头文件和宏定义可以提供一些常用的功能和数据类型,方便在代码中使用。如果你有其他问题,请继续提问。
相关推荐
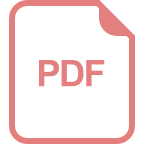
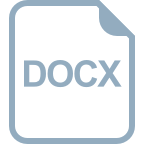
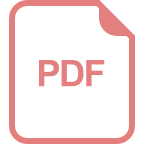












