设计一个计算链串中最小字符出现的次数的算法,使用暴力枚举
时间: 2024-10-11 16:18:02 浏览: 23
要设计一个计算链串中最小字符出现的次数的算法,你可以采用暴力枚举的方法,遍历整个链表并统计每个字符出现的次数。这里我将提供一个简单的 Java 实现步骤:
首先,我们需要定义一个链表节点(Node)结构:
```java
public class ListNode {
int val;
ListNode next;
public ListNode(int val) {
this.val = val;
}
}
```
然后,我们可以创建一个辅助方法来获取链表中的所有字符以及它们的出现次数:
```java
public static Map<Character, Integer> getCharsAndCounts(ListNode head) {
Map<Character, Integer> counts = new HashMap<>();
ListNode currentNode = head;
while (currentNode != null) {
char currentChar = (char) currentNode.val;
counts.put(currentChar, counts.getOrDefault(currentChar, 0) + 1);
currentNode = currentNode.next;
}
return counts;
}
```
接下来,我们可以遍历这个映射,找到出现次数最少的字符及其对应的次数:
```java
public static int minCharCount(Map<Character, Integer> counts) {
int minCount = Integer.MAX_VALUE;
char minChar = '\uFFFF'; // 假设没有字符出现
for (Map.Entry<Character, Integer> entry : counts.entrySet()) {
if (entry.getValue() < minCount) {
minCount = entry.getValue();
minChar = entry.getKey();
}
}
return minCount;
}
// 主函数调用
public static void main(String[] args) {
// 假设你已经有了一个链表头节点
ListNode head = ...;
// 获取字符计数
Map<Character, Integer> counts = getCharsAndCounts(head);
// 计算最小字符出现次数
int minCharAppearanceCount = minCharCount(counts);
System.out.println("最小字符出现次数: " + minCharAppearanceCount);
}
```
这个算法的时间复杂度是 O(n),其中 n 是链表的长度,因为它需要遍历链表一次。空间复杂度也是 O(1),因为只存储了字符和其出现次数。
阅读全文
相关推荐
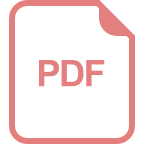
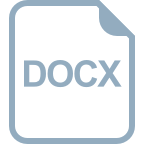
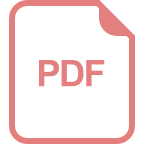
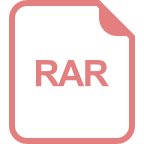
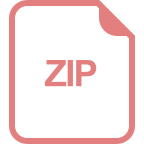
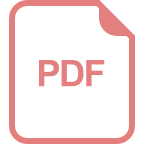
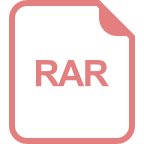
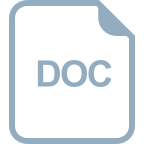
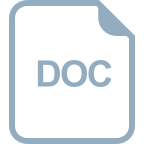
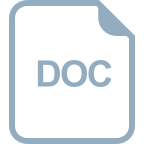
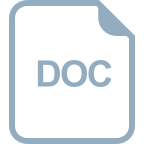
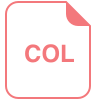
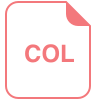
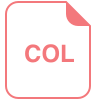


