如何在C#中将图片文件打包到可执行程序中?
时间: 2024-09-08 08:03:08 浏览: 121
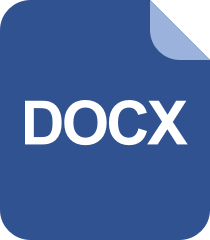
C# Winform打包和部署
在C#中,可以使用`System.IO.Compression`命名空间下的`ZipFile`类来将图片文件打包到可执行程序中。以下是简单的步骤:
1. 添加必要的命名空间引用:
```csharp
using System.IO;
using System.IO.Compression;
```
2. 定义一个方法来创建压缩包,包含图片文件:
```csharp
public static void PackImageIntoExe(string inputFile, string outputExe, string imgPath)
{
// 检查输入文件是否存在
if (!File.Exists(inputFile))
throw new FileNotFoundException($"Input file {inputFile} not found.");
// 获取图片路径相对于可执行文件的位置
string relativeImagePath = Path.Combine(imgPath, Path.GetFileName(inputFile));
// 创建一个新的zip文件流
using (FileStream zipStream = File.Create(outputExe))
using (ZipArchive archive = ZipArchive.Create(zipStream, ZipArchiveMode.Update))
{
// 添加图片文件到压缩包
ZipArchiveEntry entry = archive.CreateEntry(relativeImagePath);
byte[] buffer = File.ReadAllBytes(inputFile);
entry.Content = new MemoryStream(buffer);
}
}
```
3. 调用该方法并传入图片文件路径、原始可执行文件名以及包含图片资源的目录路径:
```csharp
string imageFilePath = "path_to_your_image.jpg";
string exeNameWithoutExtension = "YourExeName.exe"; // 不包括扩展名
PackImageIntoExe(exeNameWithoutExtension + ".dll", exeNameWithoutExtension, @"ImagesFolder\"); // ImagesFolder是你存放图片的相对路径
```
这样,图片就会被隐式地添加到可执行文件中。
阅读全文
相关推荐
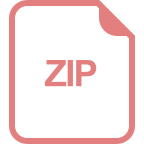
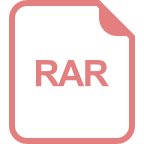
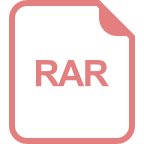
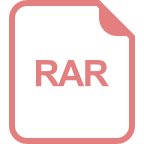
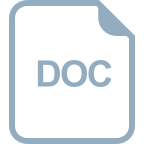
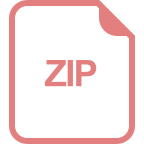
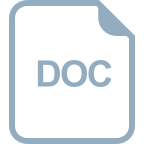
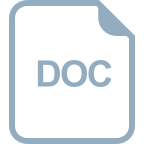
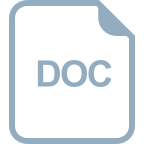
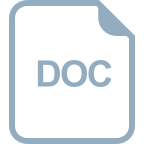
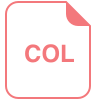
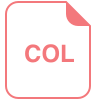
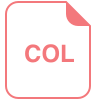
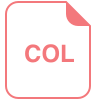
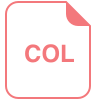

