VRP贪心算法的c++代码
时间: 2024-11-06 16:12:46 浏览: 0
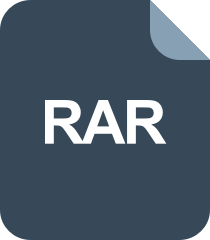
算法(c++)——多处最优服务次序问题.rar
VRP(Vehicle Routing Problem,车辆路线问题)是一种经典的优化问题,其中涉及到如何最小化送货车辆的总行驶距离,以便满足给定的客户配送需求。贪心算法是解决这类问题的一种启发式方法,它通过每一步局部最优决策试图达到全局最优解。
下面是一个简单的VRP贪心算法的C++代码示例,这里我们假设有一个基本的VRP模型,包括车辆、客户列表以及一个函数用于计算两个位置之间的距离:
```cpp
#include <iostream>
#include <vector>
#include <queue>
// 客户类,包含经纬度信息
class Customer {
public:
double x, y;
Customer(double x_, double y_) : x(x_), y(y_) {}
};
// 计算两点间的距离
double distance(Customer a, Customer b) {
return std::sqrt(std::pow(a.x - b.x, 2) + std::pow(a.y - b.y, 2));
}
// 贪心选择策略
std::vector<int> greedy_algorithm(const std::vector<Customer>& customers, int vehicle_capacity) {
std::priority_queue<std::pair<double, int>, std::vector<std::pair<double, int>>, std::greater<std::pair<double, int>>> pq;
for (int i = 0; i < customers.size(); ++i)
pq.push({distance(customers[0], customers[i]), i});
std::vector<int> route(vehicle_capacity, -1);
while (!pq.empty() && route.back() != vehicle_capacity - 1) { // 避免重复访问最后一个已添加的客户
int next_customer_id = pq.top().second;
route.push_back(next_customer_id);
pq.pop();
if (route.back() != vehicle_capacity - 1) {
for (int i = 0; i < customers.size(); ++i) {
if (customers[i].id == route.front()) {
route.erase(route.begin());
break;
}
pq.push({distance(customers[next_customer_id], customers[i]), i});
}
}
}
return route;
}
int main() {
// 示例客户数据
std::vector<Customer> customers = {/*...*/};
int vehicle_capacity = /*...*/;
auto route = greedy_algorithm(customers, vehicle_capacity);
for (int customer_id : route) {
std::cout << "Customer " << customer_id << " is in the route.\n";
}
return 0;
}
```
这个示例代码仅作为入门级讲解,实际应用中可能需要更复杂的数据结构和算法来处理更复杂的约束和需求。注意这并不是完整的VRP解决方案,因为它缺乏循环回路检测和优化等关键步骤。
阅读全文
相关推荐
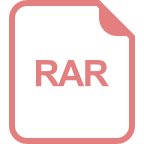
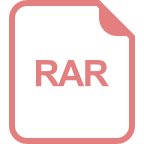
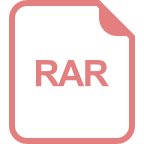
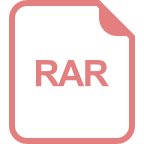
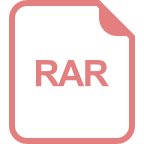

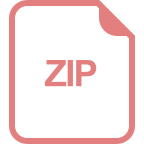
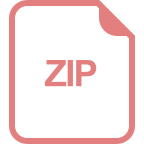
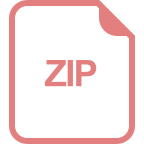
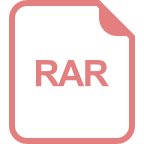
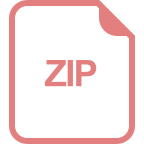
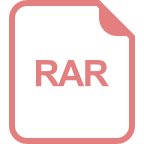