Unity文字颜色循环
时间: 2024-09-27 12:17:21 浏览: 45
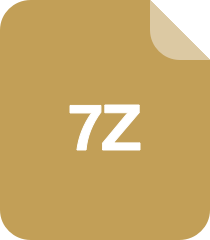
dotween pro 1.0.244 .unitypackage
Unity是一个强大的游戏引擎,并非专门用于文本颜色循环的工具,但它确实可以实现这样的效果。在Unity中,如果你想让游戏内的文字颜色随着游戏进程或时间动态变化,你可以通过以下步骤来实现:
1. 创建UI Text组件:在场景中添加Text UI元素,这是显示文本的地方。
2. 创建脚本:编写C#或其他支持的语言的脚本来控制文字颜色的变化。例如,你可以创建一个周期函数,每隔一段时间改变Text的颜色。
```csharp
public class TextColorCycle : MonoBehaviour
{
public Color[] colors; // 颜色数组,比如[Color.red, Color.green, Color.blue]
private int currentIndex = 0;
void Update()
{
if (currentIndex >= colors.Length)
currentIndex = 0;
text.color = colors[currentIndex]; // text是你Text组件的引用
currentIndex++;
}
}
```
3. 设置动画:如果你想要更平滑的颜色过渡,可以使用`Color.Lerp`方法创建插值,或者使用`Colorwheel`组件配合关键帧动画。
阅读全文
相关推荐
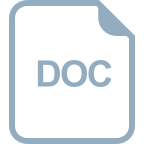
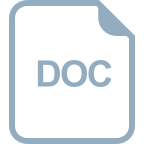
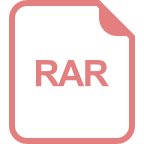
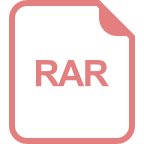


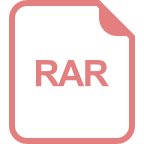
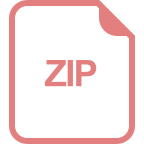
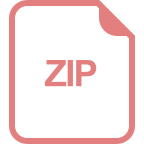
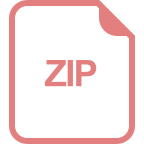
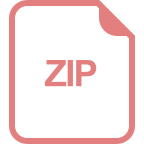
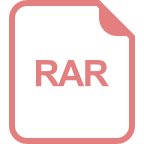
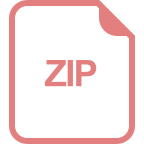
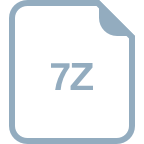



