用java1创建长度为n的带头结点的链表。
时间: 2024-12-02 09:19:10 浏览: 6
在Java中,创建一个长度为n的带头结点的单向链表通常涉及以下几个步骤:
1. 首先,你需要创建一个`Node`类来表示链表的节点,它将包含数据域(`data`)和指向下一个节点的引用(`next`)。
```java
public class Node {
int data;
Node next;
public Node(int data) {
this.data = data;
this.next = null;
}
}
```
2. 然后,你可以创建一个`LinkedList`类,其中包含一个头结点(`head`)并提供一些基本的方法如添加元素到链表尾部、获取链表长度等。
```java
public class LinkedList {
private Node head;
// 构造函数,初始化为空链表
public LinkedList() {
head = null;
}
// 添加元素到链表尾部
public void add(int data) {
if (head == null) {
head = new Node(data);
} else {
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = new Node(data);
}
}
// 获取链表长度(不包括头结点)
public int length() {
int count = 0;
Node temp = head;
while (temp != null) {
count++;
temp = temp.next;
}
return count;
}
}
```
阅读全文
相关推荐
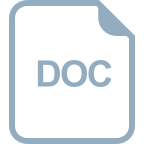
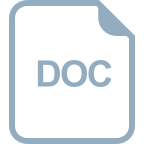
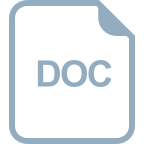
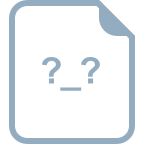



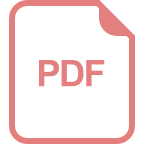
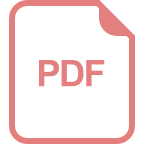
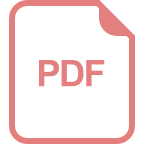
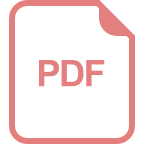
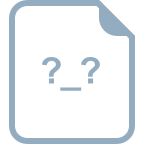
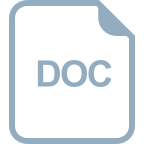


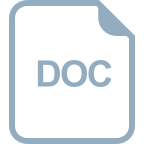
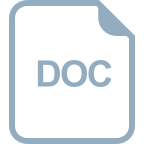