设计一个不带头结点的单链表类,要求:(1) 编写不带头结点的单链表类中的成员函数,包括求线性表的长度、插入、删除和取结点值的操作函数。(2) 设计一个测试主函数,使其实际运行来验证类中各成员函数的正确性。java语言
时间: 2024-09-15 15:12:23 浏览: 49
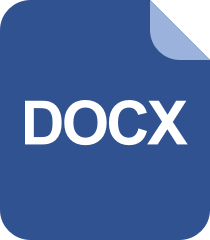
利用带头结点的单链表实现两个集合的并、交、差运算.docx
在Java中设计一个不带头结点的单链表类,可以按照以下步骤进行:
```java
public class SinglyLinkedList {
private Node current; // 当前节点
// 结点类
private static class Node {
int data;
Node next;
public Node(int data) {
this.data = data;
this.next = null;
}
}
// 成员函数
public int length() {
if (current == null) {
return 0;
} else {
int count = 1;
Node temp = current.next;
while (temp != null) {
count++;
temp = temp.next;
}
return count;
}
}
public void insert(int value) {
current = new Node(value);
if (current.next == null) { // 如果链表为空
current = current.next;
} else {
Node temp = current;
while (temp.next != null) {
temp = temp.next;
}
temp.next = current;
}
}
public void delete(int value) {
if (current == null) {
return;
}
if (current.data == value) {
if (current.next == null) { // 如果头节点就是目标节点
current = null;
} else {
current = current.next;
}
} else {
Node prev = current;
Node curr = current.next;
while (curr != null && curr.data != value) {
prev = curr;
curr = curr.next;
}
if (curr != null) {
prev.next = curr.next;
}
}
}
public int getValue(int index) {
if (index < 0 || index >= length()) {
throw new IndexOutOfBoundsException("Index out of bounds");
}
Node temp = current;
for (int i = 0; i < index; i++) {
temp = temp.next;
}
return temp.data;
}
// 测试主函数
public static void main(String[] args) {
SinglyLinkedList list = new SinglyLinkedList();
// 插入元素
list.insert(1);
list.insert(2);
list.insert(3);
System.out.println("Length: " + list.length()); // 应该输出3
// 删除元素并检查
list.delete(2);
System.out.println("After deleting 2: " + list.getValue(0)); // 应该输出1
try {
System.out.println("Value at index 3: " + list.getValue(3)); // 应该抛出异常
} catch (IndexOutOfBoundsException e) {
System.out.println(e.getMessage());
}
}
}
```
在这个例子中,我们创建了一个`SinglyLinkedList`类,包含了一些基本操作的函数,如获取长度、插入节点、删除节点以及获取指定索引处的值。测试主函数展示了如何使用这些函数。注意,这个例子假设所有的插入、删除和访问都是在有效索引范围内进行的。如果需要处理边界情况,可以在相应函数内部添加更多的错误检查。
阅读全文
相关推荐
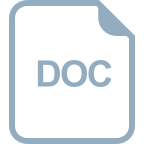



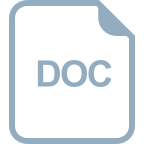
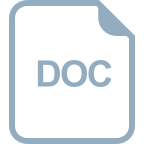
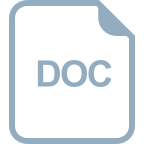








