数据结构线性表,设计一个算法,求一个不带头结点的单链表中的结点个数
时间: 2024-09-29 15:06:26 浏览: 40
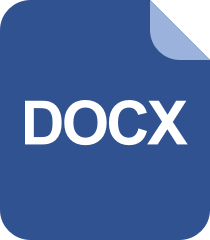
西南交通大学-数据结构实验报告-两个线性表求交集
数据结构线性表中的单链表是一个动态的数据结构,每个节点包含数据域和指向下一个节点的指针。要计算一个不带头结点的单链表中的节点个数,可以使用迭代或递归的方式。这里提供一种简单的迭代算法:
```python
def count_nodes(head):
if head is None: # 如果头节点为空,链表为空,返回0
return 0
else:
current = head # 初始化当前节点为头节点
count = 1 # 初始化计数器为1
while current.next is not None: # 当前节点不为空时继续遍历
current = current.next # 移动到下一个节点
count += 1 # 每次访问一个节点,计数加一
return count # 返回节点总数
# 示例:
# head -> 1 -> 2 -> 3 -> 4 -> None
head = Node(1) # 假设Node是一个链表节点类,有data属性和next属性
head.next = Node(2)
head.next.next = Node(3)
head.next.next.next = Node(4)
print(count_nodes(head)) # 输出:4
```
阅读全文
相关推荐
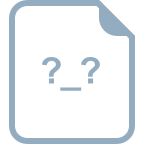
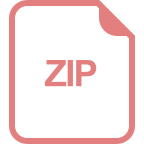
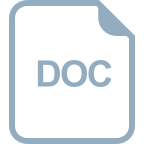









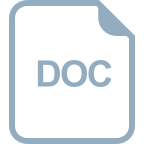



