C语言求n内素数的个数
时间: 2024-12-16 08:20:09 浏览: 10
在C语言中,计算一定范围内(比如n以内)的素数个数通常会采用筛法算法,其中最常见的是埃拉托斯特尼筛法(Sieve of Eratosthenes)。下面是基本的步骤:
1. 创建一个大小为n+1的布尔数组isPrime,初始值都设为true,表示所有数字都是素数。
2. 从2开始,遍历到sqrt(n),对于每个素数i,将它的倍数(除了它本身)标记为合数(isPrime[i*j]=false),因为大于i的i的倍数肯定不是素数了。
3. 遍历结束后,isPrime数组中剩下的true元素对应的索引就是n以内的素数。
例如,以下是简单的C语言代码实现:
```c
#include <stdio.h>
#include <stdbool.h>
#include <math.h>
int countPrimes(int n) {
bool isPrime[n + 1];
memset(isPrime, true, sizeof(isPrime));
for (int i = 2; i * i <= n; i++) {
if (isPrime[i]) {
for (int j = i * i; j <= n; j += i)
isPrime[j] = false;
}
}
int primeCount = 0;
for (int i = 2; i <= n; i++)
if (isPrime[i])
primeCount++;
return primeCount;
}
int main() {
int n;
printf("Enter the range (n): ");
scanf("%d", &n);
int primes = countPrimes(n);
printf("Number of primes less than or equal to %d is %d.\n", n, primes);
return 0;
}
```
阅读全文
相关推荐
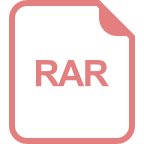
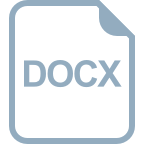















