def spiral_search(center, radius, step): x, y = center yield x, y for r in range(1, radius + step, step): for i, (dx, dy) in enumerate(((1, 0), (0, 1), (-1, 0), (0, -1))): for j in range(r - (i % 2)): x += dx y += dy yield x, y output_path = 'C:\yingxiang\mask.png' def extract_building_shadow(image, dsm, ground_spacing, radius): shadow_mask = np.zeros_like(image, dtype=np.bool) for i in range(0, image.shape[0], ground_spacing): for j in range(0, min(image.shape[1], i+ground_spacing), ground_spacing): if not np.any(shadow_mask[i, j]): center = (i, j) ground_height = dsm[i, j] for x, y in spiral_search(center, radius, ground_spacing): if x < 0 or x >= image.shape[0] or y < 0 or y >= image.shape[1]: continue if np.any(shadow_mask[x, y:]): continue height = dsm[x, y] if height > ground_height: shadow_mask[x, y] = True elif height == ground_height: if np.linalg.norm(np.array([x, y]) - np.array(center)) < \ np.linalg.norm(np.array([i, j]) - np.array(center)): shadow_mask[x, y] = True cv2.imwrite(output_path, shadow_mask.astype(np.uint8) * 255) return shadow_mask image = cv2.imread('C:\yingxiang\DJI_20230322140516_0026_V.JPG') dsm_path = 'C:/sanwei/jianmo/Productions/Production_2/Production_2_DSM_part_2_2.tif' dsm_dataset = gdal.Open(dsm_path) extract_building_shadow(image, dsm_dataset.ReadAsArray(), 10, 10)在这段代码中,想要螺旋扫描是针对dsm图像进行扫描应该怎么改
时间: 2024-03-26 10:40:38 浏览: 21
要想螺旋扫描是针对dsm图像进行扫描,需要将参数中的center改为dsm图像中的像素坐标,即将以下这行代码:
```
center = (i, j)
```
修改为:
```
center = (i, j, dsm[i, j])
```
然后在spiral_search函数中,需要在yield语句前加上z,表示第三个维度,即高度:
```
x, y, z = center
yield x, y, z
```
然后在循环体中,仅需要在height的赋值语句中,将dsm数组的第三维度也加上即可:
```
height = dsm[x, y, z]
```
最后在调用spiral_search函数时,需要将第一个参数改成三元组,即(dsm中某个像素的行号, dsm中某个像素的列号, 0)。
相关问题
def spiral_search(center, radius, step): x, y = center yield x, y for r in range(1, radius + step, step): for i, (dx, dy) in enumerate(((1, 0), (0, 1), (-1, 0), (0, -1))): for j in range(r - (i % 2)): x += dx y += dy yield x, y这段代码是什么意思
这段代码是一个生成器函数,用于在给定中心点周围生成一系列坐标点。它实现了一种螺旋搜索算法,该算法将半径内的每个像素点遍历一遍。该算法从中心点开始,并沿着水平、竖直、水平、竖直的方向顺序移动。然后,它沿着螺旋路径向外扩散,每次移动一个步长。在每个步骤中,生成器函数先返回当前坐标点(即yield x, y),然后计算下一个坐标点并继续迭代,直到达到指定的半径。最终,该函数将返回一系列坐标点,这些点组成了从给定中心点开始的螺旋路径。
def extract_building_shadow(image, dsm, ground_spacing, radius): shadow_mask = np.zeros_like(image, dtype=np.bool) for i in range(0, image.shape[0], ground_spacing): for j in range(0, image.shape[1], ground_spacing): if not np.any(shadow_mask[i, j]): center = (i, j) ground_height = dsm[i, j] for x, y in spiral_search(center, radius, ground_spacing): if x < 0 or x >= image.shape[0] or y < 0 or y >= image.shape[1]: continue if np.any(shadow_mask[x, y:]): continue height = dsm[x, y] if height > ground_height: shadow_mask[x, y] = True elif height == ground_height: if np.linalg.norm(np.array([x, y]) - np.array(center)) < \ np.linalg.norm(np.array([i, j]) - np.array(center)): shadow_mask[x, y] = True cv2.imwrite(output_path, shadow_mask.astype(np.uint8) * 255) return shadow_mask image = cv2.imread('C:\yingxiang\DJI_20230322140516_0026_V.JPG') dsm_path = 'C:/sanwei/jianmo/Productions/Production_2/Production_2_DSM_part_2_2.tif' dsm_dataset = gdal.Open(dsm_path) output_path = 'C:\yingxiang\mask.png'这段代码为什么运行后不能将掩膜结果保存
这可能是由于在保存 PNG 文件之前,尝试在代码中访问 `output_path` 变量,而此时该变量尚未定义。您可以将 `output_path` 变量的定义移动到函数之前,以确保在访问变量之前它已经存在。例如:
```
output_path = 'C:\yingxiang\mask.png'
def extract_building_shadow(image, dsm, ground_spacing, radius):
shadow_mask = np.zeros_like(image, dtype=np.bool)
for i in range(0, image.shape[0], ground_spacing):
for j in range(0, image.shape[1], ground_spacing):
if not np.any(shadow_mask[i, j]):
center = (i, j)
ground_height = dsm[i, j]
for x, y in spiral_search(center, radius, ground_spacing):
if x < 0 or x >= image.shape[0] or y < 0 or y >= image.shape[1]:
continue
if np.any(shadow_mask[x, y:]):
continue
height = dsm[x, y]
if height > ground_height:
shadow_mask[x, y] = True
elif height == ground_height:
if np.linalg.norm(np.array([x, y]) - np.array(center)) < \
np.linalg.norm(np.array([i, j]) - np.array(center)):
shadow_mask[x, y] = True
cv2.imwrite(output_path, shadow_mask.astype(np.uint8) * 255)
return shadow_mask
image = cv2.imread('C:\yingxiang\DJI_20230322140516_0026_V.JPG')
dsm_path = 'C:/sanwei/jianmo/Productions/Production_2/Production_2_DSM_part_2_2.tif'
dsm_dataset = gdal.Open(dsm_path)
# 调用函数
extract_building_shadow(image, dsm_dataset.ReadAsArray(), 10, 10)
```
请注意,此代码还将 `dsm` 数据集转换为数组,并将其传递给函数。
相关推荐
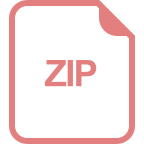
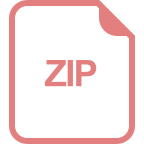
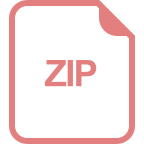




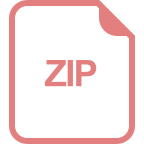
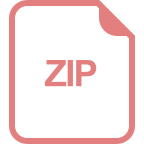
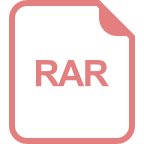
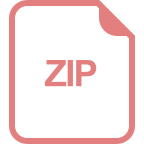
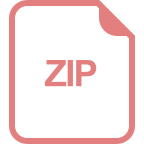
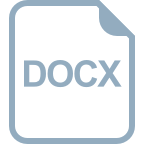
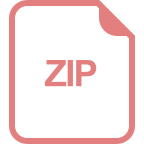