// 设置OSS访问信息 String accessKeyId = "your_access_key_id" String accessKeySecret = "your_access_key_secret" String endpoint = "your_oss_endpoint" String bucketName = "your_bucket_name" // 设置下载的文件夹路径 String ossFolder = "your_oss_folder_path" String localFolder = "your_local_folder_path" // 创建OSS客户端 def ossClient = new com.aliyun.oss.OSSClientBuilder().build(endpoint, accessKeyId, accessKeySecret) // 列出指定前缀的所有文件 def fileList = ossClient.listObjects(bucketName, ossFolder).getObjectSummaries() // 创建本地文件夹 new File(localFolder).mkdirs() // 下载文件夹中的所有文件 fileList.each { String ossFilePath = it.getKey() String localFilePath = localFolder + "/" + ossFilePath.substring(ossFolder.length() + 1) if (!it.isDirectory()) { // 下载文件 def object = ossClient.getObject(bucketName, ossFilePath) def inputStream = object.getObjectContent() def outputStream = new FileOutputStream(localFilePath) byte[] buffer = new byte[1024] int bytesRead while ((bytesRead = inputStream.read(buffer)) != -1) { outputStream.write(buffer, 0, bytesRead) } outputStream.close() inputStream.close() } }这段代码报错:isDirectory() is applicable for argument types:() values:[]
时间: 2024-04-11 09:32:05 浏览: 81
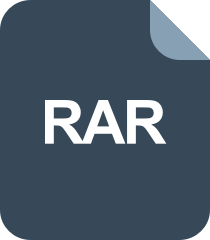
OssService_rockymtv_阿里云_ossservice_OSS_
这个错误是因为`fileList`是一个空列表,没有任何文件。因此,你需要在使用`fileList`之前,确保你的OSS存储桶中有文件。你可以在代码执行之前,检查存储桶中是否有文件,或者使用`try-catch`来处理这个错误。以下是一个示例代码:
```groovy
// 列出指定前缀的所有文件
def fileList = []
try {
fileList = ossClient.listObjects(bucketName, ossFolder).getObjectSummaries()
} catch (Exception e) {
// 处理错误,如打印错误信息等
}
// 创建本地文件夹
new File(localFolder).mkdirs()
// 下载文件夹中的所有文件
fileList.each {
String ossFilePath = it.getKey()
String localFilePath = localFolder + "/" + ossFilePath.substring(ossFolder.length() + 1)
if (!it.isDirectory()) {
// 下载文件
def object = ossClient.getObject(bucketName, ossFilePath)
def inputStream = object.getObjectContent()
def outputStream = new FileOutputStream(localFilePath)
byte[] buffer = new byte[1024]
int bytesRead
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead)
}
outputStream.close()
inputStream.close()
}
}
```
在这个示例中,我们使用了一个`try-catch`块来捕获可能的错误,并在出现错误时处理它们。你可以根据实际情况自定义错误处理逻辑。
阅读全文
相关推荐
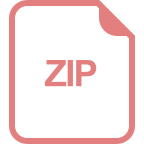
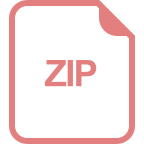


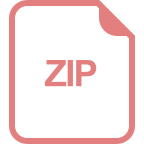












