如何在Python中运用ARIMA模型进行时间序列的平稳性检验并预测数据走势?请提供具体的代码实现。
时间: 2024-12-03 14:30:04 浏览: 16
在使用ARIMA模型进行时间序列预测时,平稳性检验是关键步骤之一。首先,你需要对数据进行必要的预处理,如数据清洗和转换。接着,使用ADF检验来确定时间序列是否平稳,如果需要,通过差分来达到平稳状态。然后,根据ACF和PACF图来辅助确定ARIMA模型的参数。在Python中,可以利用`statsmodels`库来进行ADF检验和ARIMA模型的拟合。以下是一个具体的代码示例来说明这一过程:
参考资源链接:[Python ARIMA模型进行时间序列预测](https://wenku.csdn.net/doc/2foit3j3gt?spm=1055.2569.3001.10343)
首先,导入必要的库:
```python
import pandas as pd
from statsmodels.tsa.stattools import adfuller
from statsmodels.tsa.arima.model import ARIMA
import matplotlib.pyplot as plt
```
然后,加载数据并设置索引:
```python
df = pd.read_csv('timeseries_data.csv')
df['date'] = pd.to_datetime(df['date'])
df.set_index('date', inplace=True)
```
对数据进行平稳性检验:
```python
result = adfuller(df['value'])
print('ADF Statistic: %f' % result[0])
print('p-value: %f' % result[1])
```
根据ADF检验结果进行差分,如果数据非平稳,可以进行一次或多次差分:
```python
if result[1] > 0.05: # 假设p值大于0.05则认为是非平稳
df['diff'] = df['value'].diff().dropna()
else:
df['diff'] = df['value']
```
再次进行ADF检验确认数据的平稳性:
```python
result_diff = adfuller(df['diff'].dropna())
print('ADF Statistic: %f' % result_diff[0])
print('p-value: %f' % result_diff[1])
```
确定ARIMA模型参数后,对模型进行拟合:
```python
# 假设通过ACF和PACF图确定ARIMA(1,1,1)模型
model = ARIMA(df['diff'], order=(1, 1, 1))
model_fit = model.fit()
```
进行预测并绘制结果:
```python
forecast = model_fit.forecast(steps=12) # 预测未来12个时间点的数据
# 绘制预测结果和实际数据对比图
plt.figure(figsize=(12, 6))
plt.plot(df['value'], label='Actual')
plt.plot(pd.concat([df['value'], forecast], axis=1).iloc[-12:], label='Forecast')
plt.legend()
plt.show()
```
以上代码展示了如何在Python中使用ARIMA模型进行平稳性检验并预测未来数据走势。实际应用中,可能需要根据数据特性调整ARIMA模型的参数,并对预测结果进行深入分析和评估。
参考资源链接:[Python ARIMA模型进行时间序列预测](https://wenku.csdn.net/doc/2foit3j3gt?spm=1055.2569.3001.10343)
阅读全文
相关推荐
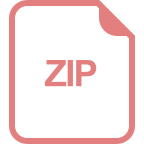
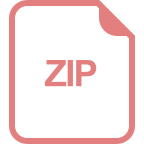
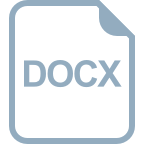

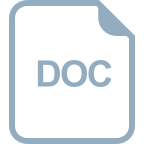
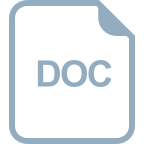




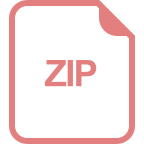
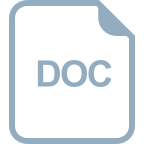
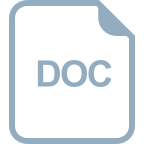
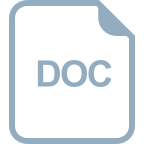




