python基于rsa的非对称加密体制,要求有,p,q不需要大素数,普通素数即可,且p,q,e要求为可输入数字,明文信息为任意可输入数字
时间: 2023-10-14 20:06:01 浏览: 90
首先,需要明确一点,RSA算法的安全性与p、q的大小有关系,因此p、q选取过小的素数会降低加密的安全性。
以下是基于RSA算法的非对称加密体制的代码实现:
```python
import random
def gcd(a, b):
while b:
a, b = b, a % b
return a
def egcd(a, b):
if a == 0:
return (b, 0, 1)
else:
g, y, x = egcd(b % a, a)
return (g, x - (b // a) * y, y)
def mod_inv(a, m):
g, x, y = egcd(a, m)
if g != 1:
raise Exception('modular inverse does not exist')
else:
return x % m
def is_prime(n):
if n <= 1:
return False
elif n <= 3:
return True
elif n % 2 == 0 or n % 3 == 0:
return False
i = 5
while i ** 2 <= n:
if n % i == 0 or n % (i+2) == 0:
return False
i += 6
return True
def generate_keypair(p, q, e):
if not (is_prime(p) and is_prime(q)):
raise Exception('p and q must be prime')
elif p == q:
raise Exception('p and q cannot be equal')
n = p * q
phi = (p-1) * (q-1)
if gcd(phi, e) != 1:
raise Exception('e must be coprime to phi(n)')
d = mod_inv(e, phi)
return ((n, e), (n, d))
def encrypt(pk, plaintext):
n, e = pk
cipher = pow(plaintext, e, n)
return cipher
def decrypt(pk, ciphertext):
n, d = pk
plaintext = pow(ciphertext, d, n)
return plaintext
# 输入p、q、e、明文信息
p = int(input("Enter a prime number (p): "))
q = int(input("Enter another prime number (q): "))
e = int(input("Enter a value for e: "))
plaintext = int(input("Enter the plaintext: "))
# 生成公钥和私钥
public_key, private_key = generate_keypair(p, q, e)
# 加密
ciphertext = encrypt(public_key, plaintext)
print("The ciphertext is: ", ciphertext)
# 解密
decrypted = decrypt(private_key, ciphertext)
print("The decrypted plaintext is: ", decrypted)
```
需要注意的是,输入的p、q、e应该为素数,否则会抛出异常。输入的明文信息需要在n范围内,否则解密时会出错。
阅读全文
相关推荐


















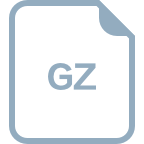
