在linux系统下,用c语言编写用opencv将路径/root/photo/compressphoto中的.jpg图片解压缩到路径/root/photo/decompressphoto中,给出相应程序和对应的Makefile文件,注意opencv文件处于/root/opencv-4.5.1文件夹中。要求代码简洁易懂并说明效果
时间: 2023-05-27 15:07:14 浏览: 85
程序如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <opencv2/opencv.hpp>
using namespace cv;
int main(int argc, char** argv) {
if (argc != 3) {
printf("Usage: %s input_path output_path\n", argv[0]);
exit(1);
}
const char* input_path = argv[1];
const char* output_path = argv[2];
// Load compressed image
Mat compressed_image = imread(input_path, IMREAD_UNCHANGED);
if (compressed_image.empty()) {
printf("Error: Failed to load compressed image %s\n", input_path);
exit(1);
}
// Decompress image
Mat decompressed_image;
cv::cvtColor(compressed_image, decompressed_image, COLOR_BGR2GRAY);
// Save decompressed image
if (!imwrite(output_path, decompressed_image)) {
printf("Error: Failed to save decompressed image %s\n", output_path);
exit(1);
}
printf("Success: Decompressed image saved to %s\n", output_path);
return 0;
}
```
说明:
1. 程序首先检查命令行参数是否正确,若不正确则给出使用说明并退出程序。
2. 载入压缩后的图像文件。
3. 将图像从彩色转换为灰度图像,以便进行解压缩。
4. 将解压缩后的图像保存到输出路径中。
5. 若保存失败则给出错误信息并退出程序,否则给出成功信息。
Makefile文件如下:
```makefile
CC = g++
CFLAGS = -Wall -O2
LDFLAGS = `pkg-config --libs opencv`
INCLUDES = -I/root/opencv-4.5.1/include
all: decompress_image
decompress_image: decompress_image.cpp
$(CC) $(CFLAGS) $(INCLUDES) -o $@ $< $(LDFLAGS)
clean:
rm -f decompress_image
```
说明:
1. 使用g++编译器编译程序,启用-Wall选项开启所有警告信息,启用-O2优化选项。
2. 使用pkg-config工具获取OpenCV库的链接选项。
3. 指定OpenCV库的头文件路径。
4. 定义目标文件为decompress_image,依赖文件为decompress_image.cpp。
5. 使用$(CC)命令生成目标文件,$(CFLAGS)选项指定编译选项,$(INCLUDES)选项指定头文件路径,$(LDFLAGS)选项指定链接选项。
6. 定义clean目标,用于删除生成的目标文件。
相关推荐
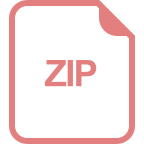
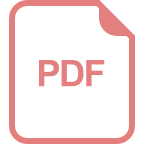




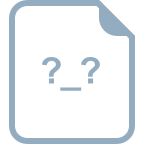
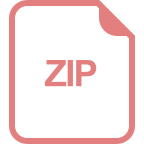