怎么在uniapp中使用vuex
时间: 2024-06-12 14:10:10 浏览: 108
在uniapp中使用vuex需要先安装vuex依赖,然后在项目中创建store文件夹,在store文件夹中创建index.js文件,然后在index.js中引入vuex并创建store对象,最后在main.js中引入store并挂载到Vue实例上即可。
示例代码如下:
1. 安装vuex依赖
```
npm install vuex --save
```
2. 创建store/index.js文件
```
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
const store = new Vuex.Store({
state: {
count: 0
},
mutations: {
increment(state) {
state.count++
}
}
})
export default store
```
3. 在main.js中引入store并挂载到Vue实例上
```
import Vue from 'vue'
import App from './App'
import store from './store'
Vue.config.productionTip = false
App.mpType = 'app'
const app = new Vue({
store,
...App
})
app.$mount()
```
相关问题
uniapp中使用vuex的步骤
在uniapp中使用Vuex的步骤如下:
1. 安装Vuex
在终端中进入项目根目录,执行以下命令安装Vuex:
```
npm install vuex --save
```
2. 创建store
在src目录下创建一个store文件夹,然后在该文件夹下创建一个index.js文件。在该文件中引入Vue和Vuex,并创建一个store实例。
示例:
```js
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
const store = new Vuex.Store({
state: {
count: 0
},
mutations: {
increment(state) {
state.count++
}
}
})
export default store
```
3. 在main.js中挂载store
在main.js中导入store,并将其挂载到Vue实例中。
示例:
```js
import Vue from 'vue'
import App from './App'
import store from './store'
Vue.config.productionTip = false
App.mpType = 'app'
const app = new Vue({
...App,
store
})
app.$mount()
```
4. 在组件中使用Vuex
在需要使用Vuex的组件中,通过$store来访问store中的状态和方法。
示例:
```js
<template>
<div>
<p>Count: {{ $store.state.count }}</p>
<button @click="$store.commit('increment')">Increment</button>
</div>
</template>
<script>
export default {
methods: {
increment() {
this.$store.commit('increment')
}
}
}
</script>
```
以上就是使用Vuex的基本步骤,根据具体需求可以在store中定义state、mutations、actions等来管理应用的状态。
uniapp如何使用vuex修改用户名称
要在Uniapp中使用Vuex修改用户名称,需要进行以下步骤:
1. 在Vuex Store中定义一个state来保存用户名称,例如:
```
state: {
userName: 'John'
},
```
2. 创建一个mutation来更新state中的userName,例如:
```
mutations: {
updateUserName(state, newName) {
state.userName = newName;
}
},
```
3. 在组件中引入Vuex,并使用mapState将state中的userName映射到组件中,例如:
```
import { mapState } from 'vuex';
export default {
computed: {
...mapState(['userName'])
}
}
```
4. 在组件中使用mapMutations将updateUserName映射到组件中,并在需要修改用户名的地方调用该mutation,例如:
```
import { mapMutations } from 'vuex';
export default {
methods: {
...mapMutations(['updateUserName']),
changeName() {
// 调用mutation更新用户名
this.updateUserName('newName');
}
}
}
```
这样就可以通过调用mutation来更新state中的userName,从而修改用户名称。
阅读全文
相关推荐
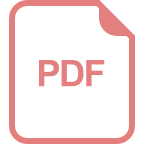
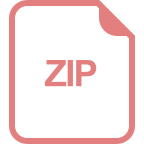
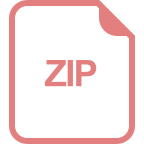













